- What does the following code print?
int x = 10;
int a = x++;
cout << a << " " << x << endl;
- 11 11
- 11 10
- 10 10
- 10 11
- What does the following code print?
int x = 10;
int a = --x;
cout << a << " " << x << endl;
- 9 9
- 9 10
- 10 10
- 10 9
- What does the following code print?
int a = 2;
int b = 3;
cout << a / b << endl;
- 0.666666
- 0.666667
- 0
- 0.000000
- 1
- What does the following code print?
int sum = 29;
int count = 10;
cout << sum / count << endl;
- 2.9
- 3
- 2
- What does the following code print?
int sum = 29;
int count = 10;
cout << (double)sum / count << endl;
- 2.9
- 3
- 2
- What does the following code print?
int sum = 29;
int count = 10;
cout << double(sum / count) << endl;
- 2.9
- 3
- 2
- What does the following code print?
double a = 2;
double b = 3;
cout << a / b << endl;
- 0.666666
- 0.666667
- 0
- 0.000000
- 1
- The variables a and b are type double and it is an error to assign integer values to them.
- What does the following code print?
cout << 3 % 4 << endl;
- 0
- 1
- 2
- 3
- 4
- 5
- What does the following code print?
cout << 5 % 4 << endl;
- 0
- 1
- 2
- 3
- 4
- 5
- What does the following code print?
cout << 5 % 5 << endl;
- 0
- 1
- 2
- 3
- 4
- 5
- What does the following code print?
cout << 5 % 6 << endl;
- 0
- 1
- 2
- 3
- 4
- 5
- m and n are integers that are greater than 0. What is the range of possible values produced by the C++ expression m % n (hint: look for a pattern in the last three questions)?
- 0 to m
- 0 to m - 1
- 0 to n
- 0 to n - 1
- 0 to m - n
- 0 to n - m
- What is the purpose of the following statement from the money.cpp example program?
pennies = pennies % 25;
- It is the number of quarters that can be converted from the remaining pennies.
- It is the number of pennies that can be converted to quarters.
- It removes from the variable pennies the number of pennies that was converted to quarters.
- A program defines a variable named x. What does the following code print?
cout << sizeof(x) << endl;
- The number of bytes of memory needed to store variable x.
- The number of bits of memory needed to store variable x.
- This is a syntax error and must be written as:
cout << sizeof x << endl;
- x and y are two double variables. Write an expression to calculate xy.
- The payment.cpp example program contains the following statement:
double payment = principal * R / (1 - pow(1 + R, -N));
Choose the best statement to describe the two sets of parentheses and the two minus signs.
- The outer set of parentheses is for grouping, the inner set of parentheses calls a function, and both minus signs are the arithmetic subtraction operator.
- The outer set of parentheses is for grouping, the inner set of parentheses calls a function, the first minus sign is the arithmetic subtraction operator, and the last minus sign is the unary negation operator.
- Both sets of parentheses are for grouping, and both minus signs represent the arithmetic subtraction operation.
- Both sets of parentheses are for grouping, the first minus sign is the arithmetic subtraction operator, and the last minus sign is the unary negation operator.
- The ftoc.cpp example program illustrates several statements that convert a temperature from Fahrenheit to Celsius. Explain what takes place when the following statement executes.
c = (f - 32) * 5 / 9;
- The statement converts the value 32 from type int to type double because f is type double, and it can only calculate the difference if the values are the same data type. It performs the subtraction operation following the conversion. Next, it converts 5 to a double and does the multiplication operation. Finally, it converts 9 to a double and does the division operation. The statement stores the result in c.
- The expression on the right-hand side of the assignment operator is evaluated first. The program evaluates the expression right-to-left, so the division operation occurs first. It converts the result, 5/9, to a double because 5/9 cannot be represented as an int. It multiplies the quotient by the difference of f and 32. The statement stores the result c.
- The subtraction operation takes place first, and the result is truncated to an int value because 32 is an int. Then the difference is multiplied by 5 and then divided by 9. The result, which may be inaccurate because of truncation, is stored in c.
- The hypot.cpp example program defines three variables: a, b, and c. Without changing the meaning of the program or the problem that it solves (so the program is still going to calculate the hypotenuse), which variable could be eliminated by rewriting the program?
- a
- b
- c
- None of the variables can be eliminated.
- The statements
cout << '\n';
and cout << endl;
seem to have the same affect. What does the second statement do that the first does not?
- It causes a linefeed to be inserted into the output stream.
- It causes a carriage return to be inserted into the output stream.
- It makes the next line of text printed display one line down and start on the left.
- It flushes the output buffer.
- A header file is incorporated into a .cpp file using the _____ directive.
- A C++ program compiles and runs but produces incorrect output. This is an example of what kind of programming error?
- A syntax error
- A logical error
- A runtime error
- A C++ program fails to compile. This is an example of what kind of programming error?
- A syntax error
- A logical error
- A runtime error
- A C++ program compiles but does not run to completion. This is an example of what kind of programming error?
- A syntax error
- A logical error
- A runtime error
- A large C++ programs fails to compile and the compiler indicates a syntax error on line 456. Which of the following statements is most accurate?
- The syntax error must be on line 456 of the program.
- The syntax error must be above line 456 of the program.
- The syntax error must be below line 456 of the program.
- The syntax error is on or above line 456 of the program.
- The syntax error is on or below line 456 of the program.
- The compiler will always correctly identify the cause of a syntax error, making a detailed knowledge of a computer programming language and its syntax unnecessary.
- True
- False
- C++ documentation provides the following description for the square root library function:
double sqrt(double x);
where x ≥ 0. Choose the best C++ implementation of the following formula (in the responses below, l0
is a lower case L-zero):
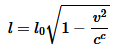
l = l0 * sqrt(1 - v*v / c*c);
l = l0 * sqrt(1 - v*v / (c*c));
l = l0(sqrt(1 - (v*v)/(c*c)));
l = l0 * sqrt(1 - pow(v,2) / pow(c,2));
- C++ documentation provides the following description for the square root library function:
double sqrt(double x);
where x ≥ 0. Choose the best C++ implementation of the following formula:
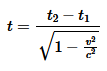
t = (t2 - t1) / sqrt(1 - pow(v,2) / pow(c,2));
t = (t2 - t1) / sqrt(1 - v*v / (c*c));
t = (t2 - t1) / sqrt(1 - v*v/c/c);
t = t2 - t1 / sqrt(1 - pow(v,2) / pow(c,2));
- A program contains two variables named x and y. Each variable is an instance of a class named Foo. Variables of type Foo may be defined, and one Foo variable may be assigned to another, but the Foo class does not support other operations. Write three C++ statements that swap the values stored in variables x and y.
C++ statement 1: __________________________
C++ statement 2: __________________________
C++ statement 3: __________________________
- To calculate
xy
, C++ provides the the power library function:
double pow(double x, double y);
Note that exponentiation or raising a number to a power has a higher precedence than multiplication. Assuming that all variables are defined and initialized, choose the best statement that translates the following formula into C++ code:
![R[(1-(x+1)(x+i) to the -(n-x) power)/(2i-1)]](../2.Core/practice/formula.png)
B = (R * ((1 - (x + 1) * (pow((1 + i), ((-1) * (n-x)))) / ((2*i) - 1)));
B = (R * ((1 - (x + 1) * (pow((1 + i), ((-1) * (n-x))))) / ((2*i) - 1)));
B = (R) * ((1 - (x + 1) * (pow((1 + i), ((-1) * (n-x))))) / ((2*i) - 1)));
B = R * ((1 - (x + 1) * pow(1 + i, -(n-x))) / (2*i - 1));
B = R * ((1 - (x + 1) * pow(1 + i, -(n-x))) / 2*i - 1);
B = (R * [(1 - (x + 1) * (pow((1 + i), ((-1) * (n-x))))) / ((2*i) - 1)]);
B = R((1 - (x + 1) * pow(1 + i, -(n-x))) / (2*i - 1));
B = R[(1 - (x + 1) * pow(1 + i, -(n-x))) / (2*i - 1)];
- To calculate
xy
, C++ provides the the power library function:
double pow(double x, double y);
Using the variables
double i1;
double i2;
double n1;
double n2;
write the C++ statement (one line) to represent the following formula:
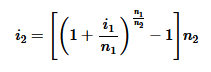
- Superscripts represent mathematical operations (exponentiation) but subscripts are just part of the variable name (replace i2 in the formula with i2 in the C++ code).
- You may not use temporary variables.
- You will need to use parentheses but try to use as few as possible.
- Choose the best elaboration to fill the blank comment in the following program:
#include <iostream>
using namespace std;
int main()
{
double f;
cout << "Enter a temperature in Fahrenheit: ";
cin >> f;
double c = 5 * (f - 32) / 9; // ____________________
cout << "The temperature in Celsius = " << c << endl;
return 0;
}
- The statement does not work because dividing by an integer causes a truncation error.
- The statement works without producing a truncation error.
- f-32 is evaluated first producing a double-value; multiplying 5 by a double produces a double, and dividing a double by 9 produces a double, so there is no truncation error.
- The assignment operator is right-associative, meaning that the statement evaluates the arithmetic expression on the right-hand side first. All arithmetic operators are left-associative, so the arithmetic expression is evaluated from left to right. The parentheses cause the statement to evaluate f-32 first. f is a double, so the 32 must be converted from an integer to a double before calculating the difference. The 5 must be converted from an integer to a double before calculating the product. Then the 9 is converted from an integer to a double before the division operation, which avoids a truncation error. Finally, the calculated value is assigned or stored in variable c.
You should carefully study the solution for this question in the answers.