Formulas
Please review: Converting Formulas to C++ Statements
Some general observations:
- Like Java, C++ does not have an exponentiation operator, so x^y ≠ xy. To raise a number to a power, you must either multiply it by itself or use pow(x,y)
- You can often use more parentheses than are needed, but too many parentheses:
- makes it difficult to balance opening and closing parentheses. For every opening '(' you must have a closing ')'
- can make the code more difficult to read and increases the chance of getting the parentheses in the wrong place.
- Here is an actual student answer; can you spot the parenthesis that is out of place?
i2 = ((pow(1+((i1*i1) / (n1*n1))), (n1/n2)) -1) * n2
- Here is another one; it's correct but I find it difficult to read and to understand
i2 = ((pow((1 + (i1 / n1)), (n1 / n2))) - 1) * n2
- Putting parentheses around individual variables serves no useful purpose:
i2 = (pow((1+((i1)/(n1))),((n1)/(n2)))-1)*n2;
Even for someone familiar with the specific formula, this code is very, very difficult to read.
- Functions returning a value are typed expressions, and statements using them follow the standard algebraic precedence rules. In the following examples, the red parentheses below are not needed while the black parentheses are.
- (sqrt(...))
- (pow(..., ...))
- (sqrt(...)) * x
- x + (pow(..., ...))
- (sqrt(...) + x) * y
- (pow(..., ...) - x) / y
- Mathematicians can choose between [, (, ], and ) - as long as they balance and match up - but C++ uses the square brackets for an entirely different purpose, so you can't use them for grouping
- In formulas, multiplication is implied by placing variables adjacent to each other: ab, but C++ requires the multiplication operator: a*b
- If you look up the sqrt or pow functions in any C++ documentation, they will look something like this:
double sqrt(double x);
double pow(double x, double y);
However, when you use the functions, you do not include the data types
- If you need to negate a variable (e.g., -n), you don't need to multiply by -1:
-1*n
(see the second example below). Most people find the code ‑n
more clear, less cluttered, and much easier to read
Library Functions
Documentation |
Example |
Program |
double sqrt(double x); |
sqrt(a*a + b*b); |
hypot.cpp |
double pow(double x, double y); |
p * r / (1 - pow(1 + r, -n)); |
payment.cpp |
The documentation for the library functions always shows the data type for each argument and for the return value. However, looking at the examples given in the videos and text, we can see the data type, double
in the examples above, is not included when we use or call the function in a program. (In this way, C++, Java, and Python are the same.)
Answers
- d
- a
- c; Whenever both operands of the division operator are type int, the operation takes place using integer arithmetic, so the result is of type int, and the value is truncated (not rounded).
- c
- a
- c; the division operation occurs first because it's inside the parentheses.
- b; Whenever at least one operand of the division operator is type float or type double, then the operation takes place using floating-point arithmetic, and the result is of type float or double (depending on the type of the operands). Integers assigned to double variables are automatically cast or promoted to double values.
- d; 3 / 4 = 0 with a remainder of 3.
- b; 5 / 4 = 1 with a remainder of 1.
- a; 5 / 5 = 1 with a remainder of 0.
- f; 5 / 6 = 0 with a remainder of 5.
- d
- c
- a
pow(x,y)
The question tests your understanding of the term "expression" and knowledge of the pow function. Be aware that C++ does not have an exponentiation operator; specifically, x^y DOES NOT raise x to the y power.
- b
- a
- c
- d
- #include
- b
- a
- c
- d
- b
- b or d
- a, b, or c
- Swapping Problem (1) used T to represent a generic data type. You only need to substitute Foo for T to answer the question. This pattern will work for all data types - including classes. Please note that the data type of temp must be the same type as the data specified in the problem, but the name of the temporary variable is not significant.
Foo temp = x;
x = y;
y = temp;
Once the value stored in x is copied to temp, then x can be changed without losing any data. Copy y to x; now y can be changed without losing data. Copy temp to y and temp is no longer needed.
- There is often not an exactly-right number of parentheses to use, but one of the purposes of this question is to demonstrate how hard it is to find errors when an excessive number of parentheses are used.
- Missing a closing parenthesis, which is hard to spot because of the many unnecessary parentheses used.
- This statement does work, but it’s not the best due to the excessive parentheses and the unnecessary "-1," which makes the code harder to read and understand.
- One too many opening parentheses.
- Just the right number of parentheses and all operators are correct.
- The denominator must be in parentheses due to the subtraction operation.
- Cannot use square brackets for grouping.
- Missing multiplication operation.
- Missing multiplication operation; cannot use square brackets for grouping.
-
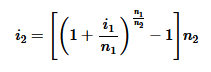
Converting the formula to C++ code may seem daunting, but taking it one step at a time makes it doable. And, sometimes it's easier to start in the middle and work your way out:
- The exponentiation problem has two parts: the base and the exponent:
- The base is:
1 + i1 / n2
- The exponent is:
n1 / n2
- Which is written as:
pow(1 + i1 / n2, n1 / n2)
- Next, do the subtraction:
pow(1 + i1 / n2, n1 / n2) - 1
- The final multiplication requires another set of parentheses and an explicit multiplication operator:
(pow(1 + i1 / n2, n1 / n2) - 1) * n2
#include <iostream>
#include <cmath>
using namespace std;
int main()
{
double n1;
double n2;
double i1;
cout << "Enter n1: ";
cin >> n1;
cout << "Enter n2: ";
cin >> n2;
cout << "Enter i1: ";
cin >> i1;
double i2 = (pow(1 + i1 / n2, n1 / n2) - 1) * n2;
cout << "i2 = " << i2 << endl;
return 0;
}
- The question is more about providing an elaboration than writing a comment. Furthermore, which elaboration is "best" depends on purpose and context, which is why more than one answer was considered correct.
Unfortunately, there is no "Goldilocks" or "just right" amount of detail to use in an elaboration. The amount of detail you include may vary depending on the situation: if you are writing the elaboration, you may want to be economical with your comments, but if you are verbalizing the elaboration to yourself or others, you can afford to be a bit more detailed. Remember, the point of elaboration is to help you understand what is taking place in the program well enough that you can use the same concepts to solve a new problem. Please see Elaboration for more information.
- The statement is incorrect.
- The statement is correct but doesn't explain what is taking place, meaning it is difficult to apply this example to a new problem.
- This statement is correct and, in many cases, is a sufficient elaboration.
- This statement is also correct and contains much more detail. This level of detail is appropriate the first time you see this problem - especially if you are verbalizing the elaboration rather than writing it.