- An array element is accessed using
- a first-in-first-out approach.
- a last-in-first-out approach.
- the dot operator.
- a member name.
- an index number.
- All the elements in an array must be the ____________ data type.
- Write a statement that defines a one-dimensional automatic array called doubleArray of type double that holds 100 elements; define the array as an automatic variable.
- The elements of a 10-element array are indexed from _______ to _______ .
- Write a statement that takes element j of array doubleArray and writes it to cout with the insertion operator.
- Write a single statement that defines an automatic int array named coins and initializes the elements to the numeric values (in pennies) of US coins: penny, nickel , dime, quarter, half-dollar, and dollar.
- When a multidimensional array is accessed, each array index is
- separated by commas.
- surrounded by brackets and separated by commas.
- separated by commas and surrounded by brackets.
- surrounded by brackets.
- C++ allows four dimensional arrays.
- true
- false
|
|
(a) | (b) |
- Choose the best statement that defines an array of type double named coefficients that matches the array illustrated in (a) above.
- double coefficients;
- double coefficients[5, 3];
- double coefficients[5][3];
- double coefficients[3, 5];
- double coefficients[3][5];
- double coefficients[4, 2];
- double coefficients[4][2];
- double coefficients[2, 4];
- double coefficients[2][4];
- Choose the best statement that stores 2 in the shaded element of the array illustrated in (b) above.
- double coefficients[4, 2] = 2;
- double coefficients[4][2] = 2;
- coefficients[4, 2] = 2;
- coefficients[4][2] = 2;
- double coefficients[2, 4] = 2;
- double coefficients[2][4] = 2;
- coefficients[2, 4] = 2;
- coefficients[2][4] = 2;
- double coefficients[3, 2] = 2;
- double coefficients[3][1] = 2;
- coefficients[3, 1] = 2;
- coefficients[3][1] = 2;
- double coefficients[1, 3] = 2;
- double coefficients[1][3] = 2;
- coefficients[1, 3] = 2;
- coefficients[1][3] = 2;
- For a two-dimensional automatic array of type int, called arr, choose a statement that defines the array and initializes the first row to 52, 27, 83; the second row to 94, 73, 49; and the third row to 3, 6, 1.
- int arr[3][3] = {{52,27,83}, {94,73,49}, {3,6,1}};
- int arr[][3] = {{52,27,83}, {94,73,49}, {3,6,1}};
- int arr[3][3] = {52,27,83,94,73,49,3,6,1}
- The name of an array, without square brackets, represents the ____ of the array.
- Given the following definitions
int scores[50];
int* my_pointer = ...; // initialized to a valid value
Which statements are valid?
- int* spointer = &scores;
- int* spointer = scores;
- scores = my_pointer;
- When an array is passed as an argument to a function,
- the function and the function caller accesses the same array.
- the function accesses a copy of the array.
- An array function argument allows data to be both passed into and out of the function.
- True
- False
- If scores is a C++ array, you can write
scores.length
to find the length of the array.
- True
- False
- Assuming that employee is the name of structure, tell what this statement defines:
employee emplist[1000];
- An array with 1000 elements of a structure named employee
- An array with 1000 elements that are pointers to structures named employee
- An pointer to an array with 1000 elements of a structure named employee
- There is insufficient information to determine what the array contains
- Assuming that employee is the name of a structure, tell what this statement defines:
employee* emplist[1000];
- An array with 1000 elements of a structure named employee
- An array with 1000 elements that are pointers to structures named employee
- An pointer to an array with 1000 elements of a structure named employee
- There is insufficient information to determine what the array employee
- Assuming that employee is the name of a structure, tell what this statement defines:
employee* emplist = new employee[1000];
- An array with 1000 elements of a structure named employee
- An array with 1000 elements that are pointers to structures named employee
- An pointer to an array with 1000 elements of a structure named employee
- There is insufficient information to determine what the array contains
- Write a statement to define an automatic array of ints, named scores, as illustrated below.
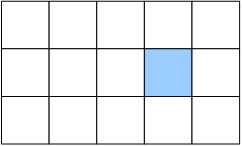
- An array of ints named scores is illustrated below. Write a statement to assign 100 to the array element indicated by the shaded region:
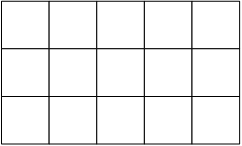
- In a stack (implemented as an array), the data item placed on the stack first is
- not given an index number.
- given the index number 0.
- the first data item to be removed.
- the last data item to be removed.
- Write a statement that defines an automatic array called manybirds that holds 50 objects of type bird.
- bird manybirds[49]; // 49 because the first element is 0
- bird manybirds[50];
- bird manybirds = new bird[49];
- bird manybirds = new bird[50];
- bird* manybirds = new bird[50];
- Choose the statement that creates a dynamic array called manybirds that holds 50 objects of type bird.
- bird manybirds[49]; // 49 because the first element is 0
- bird manybirds[50];
- bird manybirds = new bird[49];
- bird manybirds = new bird[50];
- bird* manybirds = new bird[50];
- The compiler will detect and report an error when you compile a program that accesses array element 14 in an array of size 10.
- true
- false
- The computer will always detect and report an error when you run a program that tries to access array element 14 in an array of size 10.
- true
- false
- A program defines an array as:
int a[10];
which function is able to correctly accept "a" as an argument?
- void func(int m[]) { ... }
- void func(int* m) { ... }
- void func(int m[10]) { ... }
- None of the above
- A program defines a two-dimensional array as:
int mat[5][10];
which function is able to correctly accept mat as an argument?
- void func(int m[][]) { ... }
- void func(int m[][10]) { ... }
- void func(int m[5][10]) { ... }
- void func(int m[5][]) { ... }
- None of the above
- Given an array whose size is stored in the variable size, choose the correct for-loop to use to reverse the elements in the array.
- for (int i = 0; i < size; i++)
- for (int i = 0; i < size / 2; i++)
- for (int i = 1; i <= size; i++)
- for (int i = 1; i <= size / 2; i++)
- Examine the following function and briefly (a) describe what is wrong and (b) one way to correct the error.
int* get_data()
{
int data[100];
// fill the data array
return data;
}
- If "a" is an array, it is possible in a C++ program loop through the elements of the array by:
for (int i = 0; i < a.length; i++) . . .
- True
- False
- A C++ program defines a function as
void f(foo* data, int x, int y) { . . . }
foo is the name of a fully specified structure and data is a one-dimensional array of foo objects. The variables x and y are valid indexes into data. Write the statements to swap the values stored in data[x] and data[y]. The size of data (i.e., the number of elements) is not important for this problem.
- Choose the best statement to describe the following code fragment:
char data[100];
int count = sizeof(data) / sizeof(char);
- the value saved in count is the number of elements in the array named data
- the value saved in count is meaningless because sizeof(data) is the size of the variable named data.
- Choose the best statement to describe the following code fragment:
char* data = new char[100];
int count = sizeof(data) / sizeof(char);
- the value saved in count is the number of elements in the array named data
- the value saved in count is meaningless because sizeof(data) is the size of the variable named data.
- Choose the best statement to describe the following code fragment:
void function(char* data)
{
count = sizeof(data) / sizeof(char);
}
- the value saved in count is the number of elements in the array named data
- the value saved in count is meaningless because sizeof(data) is the size of the variable named data.
- Complete the following function that copies a character array, source, to a second array, destination.
void copy(char* destination, char* source, int size)
{
}
- Write a complete function named average that takes two arguments as described below and returns a double:
- the first argument, named scores, is a one-dimension array of doubles that are test scores
- the second argument, named size, is an int that is the number of elements in the scores array
- the return value is the average or mean of all of the test scores