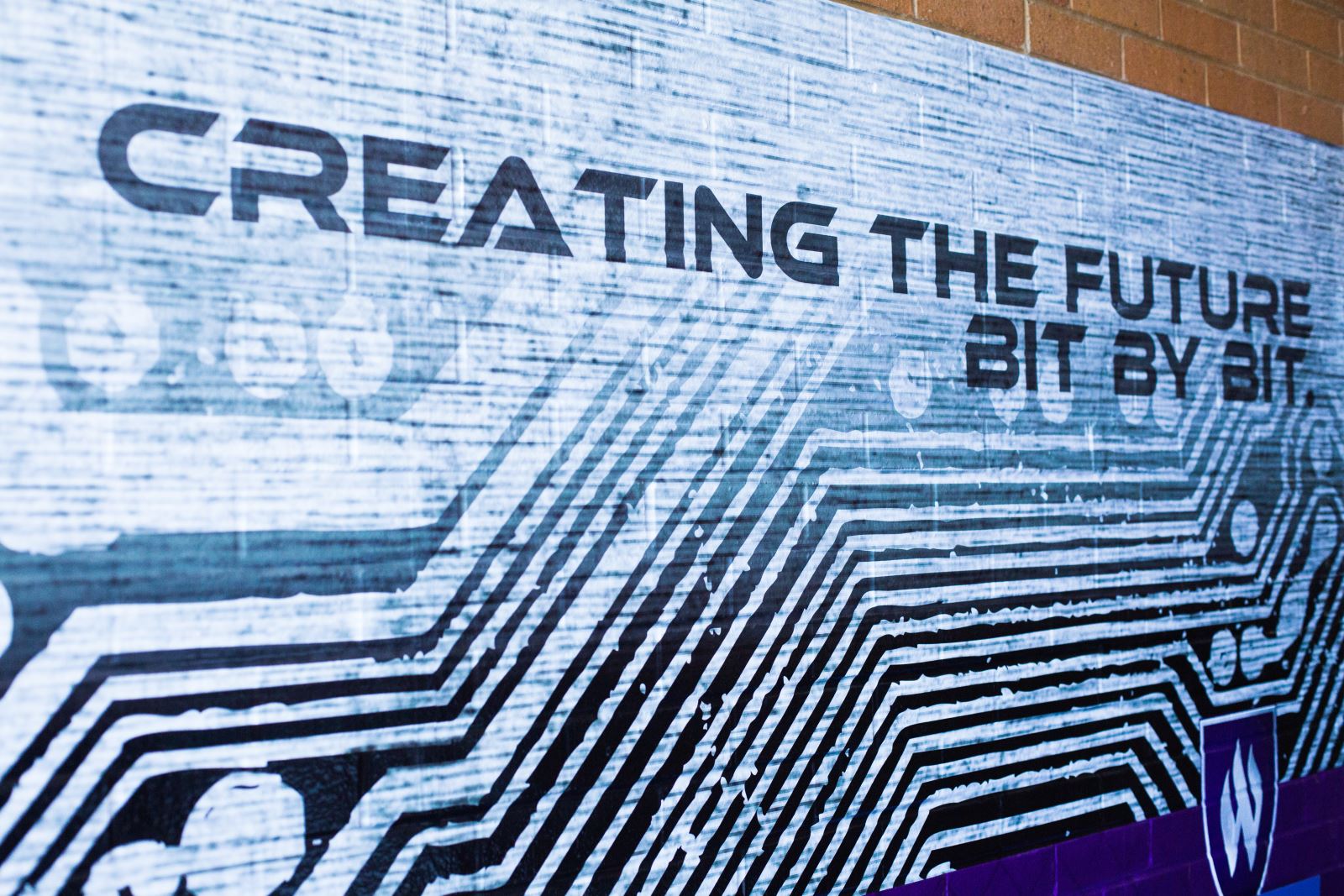
Associate Degree Assessment
- CS 1030
- CS 1400
- CS 1410
- CS 2130
- CS 2350
- CS 2420
- CS 2450
- CS 2550
- CS 2705
- CS 2810
CS 1030
CS 1400
CS 1410
- 1. Operators
- a. +, -. *, /, %, =, operation with assignment, pre/post increment/decrement, and ?:,
- b. &&, ||, !
- 2. Flow of control statements:
- a. if, if-else, if-else ladder, switch, default, and break
- b. dangling else
- c. for, while, do-while, break, continue, and test at the top versus test at the bottom
- d. null-statement
- e. block-structure
- 3. Functions and function arguments and return values
- a. pass by value, pass by reference, and pass by pointer (pass by value where the
- b. default arguments
- c. the address of operator
- d. the deference/indirection operator
- 4. Arrays and array function arguments
- 5. Structures: members/fields, the dot operator, and the arrow operator
- 6. C++ string class: string member functions including string/number conversions, C-strings and c-string functions (strlen, strcat, strcpy, strstr, strchr, strtok)
- 7. Classes and objects
- a. constructors (general, default, copy, conversion)
- b. destructors
- c. member functions
- d. friend and inline functions
- e. data members
- f. public, private, and protected
- g. dot and arrow operators
- 8. Overloaded operators
- a. friend vs. member
- b. overloaded inserter and overloaded extractor
- 9. UML (diagrams and implementation in C++)
- 10. Class relationships
- a. inheritance, association, composition, aggregation, and dependancy
- b. identifying from UML diagrams; identifying from code
- c. implementation in C++
- d. up and down casting
- e. overloading and overriding functions
- 11. Polymorphism
- 12. Exception handling
- 13. File I/O
- 14. Dynamic memory (new and delete)
- 15. Templates
- 16. File I/O
There are about 65 - 70 questions: multiple choice, short answer, and essay questions (programming).
CS 2130
Sets
- Intersection, Union, Null Set
- A ? B (symmetric difference) = A ? B -- A n B
- Addition principal - |A ? B ? C| = |A| + |B| + |C| - |A n B| - |B n C| - |A n C| + |A n B n C|
- A = U -- A (complement of A)
- Subsets
- Sets containing other sets
- Set builder notation
- Cardinality
Functions and integers
- Everywhere defined -- everything in A is used and each element in A goes to only 1 element in B
- Onto -- every element in B can be gotten to with the function ie. Range(f) = B
- 1-to-1 -- each element in B can be gotten to by at most one element in A
- Invertible -- 1-to-1 and onto
- A function is invertible if its inverse (f-1) is also a function
- g ° f -- (g ° f)(a) = g(f(a))
- f ° g -- (f ° g)(a) = f(g(a))
- Floor -- round down
- Ceiling -- round up
- LCM = 2max(a,b) * 3max(a,b) * 5max(a,b) * ... {all primes}max(a,b)
- GCD = 2min(a,b) * 3min(a,b) * 5min(a,b) * ... {all primes}min(a,b)
- Euclid's alogorithm -- d = sa + tb
- Base conversion -- alternate using / and %
Sequences
- Sequence -- order matters
- Finite -- countable, has specific start and end points
- Infinite -- has no end point. The book calls it countable, but how do you count infinity?
- Countable -- can be arranged in a list, has a start
- Uncountable -- anything not countable, an example is all real numbers between 0 and 1
- Recursive -- element depends on previous values, may be infinite, but has a specific starting point
- Explicit -- element depends only upon itself, has a specific starting point
- String -- sequence of letters, set corresponding to a sequence
- Regular Expressions -- defining a set of strings
Counting and Probability
- Multiplication principle
- Permutation -- order matters P(n,r) = n!/(n-r)!
- Combination -- order doesn't matter C(n,r) = n!/(r!(n-r)!)
- Permutation with repeats -- P(n,r) = nr
- Combination with repeats -- C(n,r) = rewrite this one as a regular combination using C(n+r-1, r)
- Event (E) -- the desired outcome or combination of outcomes
- Sample space (S) -- all possible outcomes
- Probability -- P = |E|/|S|
- Pigeonhole principle
Matrices -- Boolean and Regular
- Add, ? AND, ? OR - only exact same sizes
- Multiply -- MxN * JxK is possible only if N=J, result is a MxK matrix
- Transpose -- flip around the diagonal (first row becomes first column, etc). Is symmetric if A = AT
- Identity matrix -- binary matrix where the diagonal is all 1's, all other values are 0, is always square
- Inverse -- only computable for a 2x2 matrix (bigger can be done, but not in this class)
Propositions and logical operations
- Truth tables for logical operators
- Statement -- true or false declaration (not opinion, question, command, changing value, etc.)
Graphs
- Matix
- in-degree = number of arrows into node, number of 1's in the column
- out-degree = number of arrows out of node, number of 1's in the row
- Paths
- Cycle -- begin and end at the same vertex
- Connectivity relation showing all paths of all lengths
- Rn path of length n
- Relations
- reflexive -- R is reflexive if aRa for all a in A
- irreflexive -- R is irreflexive if aRa for all a in A
- symmetric -- aRb and bRa
- asymmetric -- aRb and bRa (a?b or both 0, diagonal is 0)
- antisymmetric -- if aRb and bRa then a=b, else aRb and bRa, or both 0
- transitive -- if aRb and bRc then aRc
- Digraph representations of relations
- Matrix representations of relations
- Graphs
- reflexive -- all nodes need a cycle of length 1
- irreflexive -- no node can have a cycle of length 1
- symmetric -- all edges go both ways, cycles of length 1 are ok
- asymmetric -- no cycle of length 1, all edges are single path
- antisymmetric -- all edges between vertices are single path, cycle of length 1 is ok
- transitive -- if there is a path of length 2 from a to c, passing through b, then there must also be a path of length 1 between a and c. If no path of length 2 exists, it is still transitive.
Growth of Function
- Big-Theta and Big-Oh Notation
Trees
- root -- first or top vertex in the tree, has a height of 0
- leaf -- bottom vertex, has 0 children
- n-tree -- all vertices have at most n children
- complete -- all vertices except leaves have the same number of children
- balanced -- height of all leaves differ by at most 1
- sub-tree -- any vertex of a tree may be partitioned off (with all children etc) to become a new tree
Grammar
- G=(V, S, v0, ->)
- G -- grammar
- V -- everything, similar to the universe
- S -- set of terminal symbols
- N -- set of non-terminal symbols
- -> - the production
Machine
- M = (S, I, F) machine or (S, I, F, s0, T) Moore machine
- S -- state set
- I -- input set
- F -- state transition function
- T -- terminal state set
- s0 -- starting state
CS 2350
Questions randomly selected on the CS 2899 exam, covers topics in XHTML 1.1, CSS, JavaScript, and JQuery.
Here is a sampling of areas to study...
- 1. Targeting INTERNAL hyperlinks.
- 2. <a> vs. <link>
- 3. Ways to create bold text (using elements and/or inline styling).
- 4. Reasons you would use tables in XHTML.
- 5. XHTML Rules (closing tags, nested elements)
- 6. <td> vs. <th>
- 7. list elements in XHTML
- 8. internal, relative, absolute and mailto hyperlinks
- 9. Image sizing and format
- 10. CSS p,h1 vs. p h1 (with space)
- 11. Form Action and Form Method
- 12. XHTML Block Elements
- 13. CSS p h1 vs. p > h1
- 14. CSS hierarchy (and override of rules)
- 15. Commenting in XHMTL
- 16. JPG vs GIF vs PNG
- 17. The 16 web safe colors (recognizable by all browsers by name)
- 18. The Input element (valid types)
- 19. img - valid attributes
- 20. table - valid attributes
- 21. The CSS Box Model
- 22. CSS Float
- 23. form - valid elements
- 24. CSS hiding underlines in hyperlinks
- 25. CSS ID's vs Class
- 26. table - valid elements
- 27. font-size: %, em, x-large, etc.
- 28. b vs strong, i vs em
- 29. input type button
- 30. XHTML inline elements
- 31. Mandatory elements in XHTML
- 32. Well-formed XHTML
- 33. Identify the correct XHTML template
- 34. Color #00ff00 vs. RGB
- 35. Table Colspan and Rowspan
- 36. Table deprecated align and bgcolor attributes
- 37. Input button vs. Input Submit - differences
- 38. The <dt> element
- 39. CSS top, right, bottom, left (the abbreviated ordering is TRBL)
- 40. Commenting in CSS
- 41. JS: window.prompt
- 42. JS: window.alert
- 43. JS DOM: radio button properties - determining which button is selected
- 44. JS DOM: text area properties - determining the content of the data entered
- 45. JS DOM: removing focus from a control
- 46. JS DOM: event triggered when a new item is selected from a list
- 47. JS DOM: checkbox properties - determining which items are selected
- 48. JS DOM: clearing a check from a checkbox object
- 49. JS: Math object method to return the largest value passed to it
- 50. JS: Escape characters in JavaScript strings
- 51. JS: Date Object - creating new date
- 52. JS: Date Object - getMonth, getDay, GetDate
- 53. JS: ToFixed()
- 54. JS: ParseFloat and ParseInt
- 55. JS: toLowerCase a
- 56. JS: = vs ==
- 57. JS: isNAN
- 58. JS: If else If
- 59. JS: switch case, break
- 60. JS: While vs. For loops
- 61. JS: break and continue
- 62. JS: concatenation of strings (or numeric)
- 63. JS: array indexing
- 64. JS: array join, splice, concat
- 65. JS: multi-dimensional arrays (general)
- 66. JS: multi-dimensional arrays and looping
- 67. JS: declaring variables
- 68. JS: creating functions
- 69. JS: function return values
- 70. JS: function parameter naming
- 71. JQuery toggle()
- 72. JQuery changing color
- 73. JQuery adding/removing classes
- 74. JQuery referencing info from a hyperlink element
- 75. JQuery selectors id vs class
- 76. JQuery selectors with multiple values
- 77. JQuery jquery-1.x.x.js vs. jquery-1.x.x.min.js
- 78. JQuery .html()
- 79. JQuery show hide
- 80. JQuery document.ready
CS 2420
CS 2450
CS 2550
Chapter 1
- Know what a table, row, and column are used for in databases
- Know what a primary key and a foreign key is
- Know which SQL statements are used for data manipulation, data definition, data control and transaction control
- Know what each form of Normalization involves. Know the keys of when data is in a given normalized form.
Chapter 2
- Know the types of commonly used data types
- Know how to write a SQL SELECT statement
- Know the purpose of the DISTINCT and UNIQUE statements
Chapter 3
- Know the different SQL Comparison Operators
- Know how to write an SQL statement checking for equality
- Know how to write and SQL statement using the greater than and less than operators
- Know how to use the BETWEEN operator
- Know how to use the IN operator
- Know how to use the LIKE operator
- Know how to use the NOT operator
- Know how to use the NULL and NOT NULL operators
- Know how to use the ORDER BY operator
- Know how to create column aliases
- Know how to add comments to your SQL statements
Chapter 4
- Know how to use the LOWER, UPPER, INITCAP functions
- Know how to use the LPAD and RPAD functions
- Know how to use the DUAL table
- Know how to use the SUBSTR function
- Know how to use the LENGTH function
- Know how to use arithmetic operators
- Know how to use the NVL function
- Know how to use the CASE function
Chapter 5
- Know how to use the TO_CHAR function to format dates
- Know how to use the TO_DATE function
Chapter 6
- Know how to use the COUNT function
- Know how to use the SUM function
- Know how to use the AVG function
- Know how to use the GROUP BY clause
- Know how to use the HAVING clause
Chapter 7
- Know how to write a table alias
- Know how to write an equijoin
- Know what a Cartesian product is
- Know how to write a three or more table join
- Know how to write a JOIN statement.
Chapter 8
- Know syntax for writing subqueries
- Know how to write subqueries
- Know how to write a subquery using the Inline View
- Know how to write a subquery where subquery is in the FROM clause
Chapter 9
- Know how to write a UNION statement
- Know how to write a MINUS operation statement
Chapter 10
- Know how to write an Outer Join statement
Chapter 11
- Know how to write an INSERT, UPDATE and DELETE statement
- Know how to write a COMMIT statement
- Know how to write a ROLLBACK statement
CS 2705
CS 2810
The 2810 exam will cover numeric conversions which include both 16-bit signed integers and 32-bit floating point numbers (IEEE-754 Single Precision). To help you focus your studies, This is a list of major ideas that may or may not be on the exam. They are in no particular order.