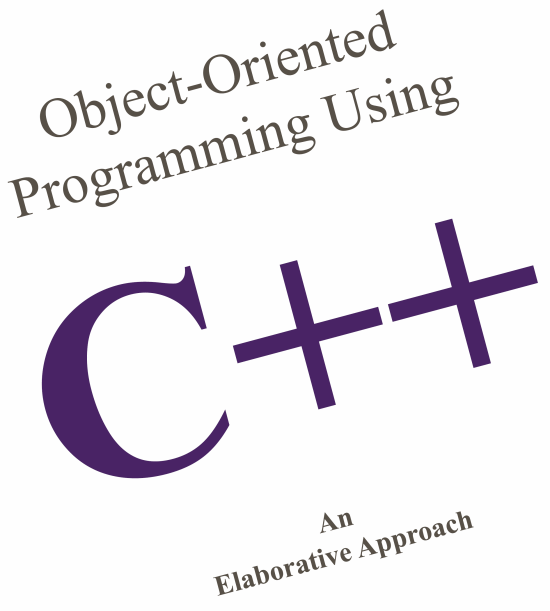
Symbols
- --
- Pre- and post-decrement decrement operators (also known as auto decrement operators). Unary operators whose operands are variables. Pre-decrement (e.g., --counter) decrements the value stored in counter then "uses" the new value; post-decrement (e.g., counter--) "uses" the current value stored in counter then decrements the stored value. See 2.8. Unusual Operators.
- !
- The logical-NOT or negation operator. A unary operator whose operand is a Boolean-valued expression. The operator nots, negates, switches, or reverses the logical value of the expression: true becomes false and false becomes true. See Logical Operators.
- !=
- A binary relational operator that compares two logical or Boolean expressions. The result of the comparison is true if the two expressions have different values, and false if the values are the same.
- %
- The modular or remainder operator. See Modular Operator Details for an illustration.
- &
- The bitwise AND operator. A binary operator that applies the AND operation to corresponding bits in the two integer operands. See Bitwise-AND for a simple example and File Access Control for an authentic one.
- &
- The address of operator. See Pointer Operators for illustrations and examples.
- &&
- The logical-AND operator. A binary operator that applies the AND operation to its two Boolean-expression operands. See Logical Operators.
- ()
- The typecast operator. A unary operator that converts its operand from one type to another. Note: the operator forms an expression with the new type, but the cast does not change the original data.
- ()
- Grouping symbols that alter the "natural" order of evaluation based on operator precedence and associativity. See Order of Operator Evaluation.
- *
- The multiplication operator. See Arithmetic Operators.
- *
- The dereference or indirection operator. See Dereferencing A Pointer for illustrations and examples.
- *
- A modifier for defining a pointer variable. See Pointer Operators.
- .
- The dot operator.
- ?:
- The conditional operator.
- []
- Depending on where they are used, the square brackets have one of two related meanings:
- A modifier for defining array variables. For example double scores[10]; defines an array named scores with a capacity of ten double variables or elements.
- The array index operator selects one element in an array. For example sum += scores[i]; selects the ith element from the scores array and adds it sum.
- ^
- The bitwise exclusive-OR (XOR) operator. A binary operator that applies the XOR operation to the corresponding bits in the two integer operands. See Bitwise-XOR for an example.
- |
- The bitwise OR operator. A binary operator that applies the OR operation to corresponding bits in the two integer operands. See Bitwise-OR for simple example and Free Space Management for an authentic one.
- ||
- The logical-OR operator. A binary operator that applies the OR operation to its two Boolean-expression operands. See Logical Operators.
- ~
- The bitwise complement operator. A unary operator that produces the one's complement of its integer operand (0's become 1's and 1's become 0's). See Bitwise Operators for details and Free Space Management for an example.
- ++
- Pre- and post-increment operators (also known as auto increment operators). Unary operators whose operands are variables. Pre-increment (e.g., ++counter) increments the value stored in counter then "uses" the new value; post-increment (e.g., counter++) "uses" the current value stored in counter then increments the stored value. See 2.8. Unusual Operators.
- ::
- See Scope resolution operator.
- <
- A relational operator whose two operands are Boolean-valued expressions. Forms a Boolean-valued expression that is true if the left hand operand is numerically less than the right hand operand.
- <=
- A relational operator whose two operands are Boolean-valued expressions. Forms a Boolean-valued expression that is true if the left hand operand is numerically less than or is equal to the right hand operand.
- <<
- The bitwise left-shift operator. Both operands, x and n, are integers: x << n shifts x n-places to the left. See Shifting Left for details and Free Space Management for examples.
- <<
- See the inserter operator.
- =
- The assignment operator is a binary operator whose left operand is a variable (or more generally an l-value) and whose right operand is a variable (or an r-value). The assignment operator forms an expression, so assignment operations can be chained together or embedded in more complex statements:
- a = b = c;
- while ((c = cin.get()) != EOF) ...
- ==
- A binary operator that tests its operands for strict equality: true if both operands are equal, false otherwise.
- >
- A relational operator whose two operands are Boolean-valued expressions. Forms a Boolean-valued expression that is true if the left hand operand is numerically greater than the right hand operand.
- >=
- A relational operator whose two operands are Boolean-valued expressions. Forms a Boolean-valued expression that is true if the left hand operand is numerically greater than or is equal to the right hand operand.
- ->
- The arrow operator.
- >>
- The bitwise right-shift operator. Both operands, x and n, are integers: x >> n shifts x n-places to the right. See Sifting Right for details and Free Space Management for examples.
- >>
- See the extractor operator.
- \'
- The escape sequence used to represent a single-quotation charter in a string or as a character constant.
- \"
- The escape sequence used to represent a double-quotation charter in a string or as a character constant.
- \a
- The escape sequence used to represent the alert (or bell) control charter in a string or as a character constant.
- \b
- The escape sequence used to represent the backspace control charter in a string or as a character constant.
- \f
- The escape sequence used to represent the form feed control charter in a string or as a character constant.
- \n
- The escape sequence used to represent the new line (line feed) character in a string or as a character constant.
- \r
- The escape sequence used to represent the carriage return character in a string or as a character constant.
- \t
- The escape sequence used to represent the horizontal tab character in a string or as a character constant.
- \xdd
- dd is replaced with two hexadecimal digits. Creates an 8-bit character; used to represent control or extended ASCII characters.
#directives
- #define
- A preprocessor directive that creates a macro. An identifier or macro name follows the directive, and a constant-valued expression usually (but not always) follows the identifier. For example:
- #define MAX 100
- #define ZEBRA
- #define SQUARE ((x) * (x))
- #elif
- A preprocessor directive read as "else if." See #ifdef.
- #else
- A preprocessor that creates a logical "else" branch in an if-then-else structure implemented with preprocessor directives. See #ifdef.
- #endif
- A preprocessor directive read as "end if." Ends or terminates a logical if-then-else structure implemented with preprocessor directives. See #ifdef.
- #ifdef
- A preprocessor directive read as "if defined." Conditionally includes code in the preprocessor's output. If "identifier1" is defined (i.e., it is in the preprocessor's symbol table), then all of the code between the #ifdef and the next conditional directive is included in the preprocessor's output. See #define (2).
#ifdef identifier1 ... #elif identifier2 ... #else ... #endif
- #ifndef
- A preprocessor directive read as "if not defined." Works like #ifdef but reverses or negates the test.
- #include
- A preprocessor directive that copies the contents of the named file into the preprocessor's output. See 1.8 The #include Directive.
- #include guard
- Also known as a macro guard. A logical structure created with preprocessor directives that ensures that a block of code, usually a header file, is included in a program exactly once. See 10.2.4. #include Guard.
- #pragma
- A preprocessor directive that introduces a "pragmatic" construct into a program. The ANSI C++ standards attempt to make conforming C++ programs portable. However, sometimes non- or less-portable code can be faster or more efficient. The #pragma directive introduces a construct that depends on the compiler, operating system, or hardware but is allowed for "pragmatic" reasons.
- #pragma once
- A preprocessor directive that replaces an #include guard. Although the #pragma once directive is not included in the ANSI standard, it is recognized by the common desktop operating systems (Windows, macOS, and Linux), and so is generally safe to use. Nevertheless, its portability is not guaranteed.
- #undef
- A preprocessor directive that undefines an identifier. It is the reverse of the #define directive.
A
- Absolute pathname (aka full pathname)
- Most general-purpose operating systems implement a hierarchical or tree-like file system with its root directory or folder at the top. An absolute pathname consists of a sequence of zero or more directories beginning at the root and following the branches downward to a specific file or directory. A path separator character, "\" for Windows systems and "/" for Unix, Linux, and macOS systems, separates each path element (directory or file name). Windows systems may also include a drive letter in an absolute path. Some examples:
- /dir1/dir2/dir3/file
- /dir1/dir2/dir3
- /file
- \dir1\dir2\dir3\file
- C:\dir1\dir2\dir3\file
- \dir1\dir2\dir3
- \file
- Abstract class
- An abstract class has at least one abstract or pure virtual function. Abstract classes cannot be instantiated (i.e., a program cannot create objects from an abstract class). Abstract classes can be superclasses, and programs can define pointer and reference variables whose type is an abstract class, allowing them to serve as the target for an upcast. Subclasses of an abstract class must override all the abstract class's functions or they inherit its abstractness. Software developers often use abstract classes to ensure that a set of subclasses implement the same function, setting the stage for polymorphism. For example, if a shape class has an abstract (i.e., a pure virtual) draw function, then all shape subclasses must have an appropriate concrete draw function.
- Abstract data type
- Abstract data types (ADT) are data types created by application and library programmers in contrast to fundamental data types that are recognized and processed directly by a compiler or interpreter. We typically characterize ADTs by the operations or services they support. For example, a stack (1) must support two operations: push and pop. In a C++ program, programmers typically create an ADT with a class or struct and #include its specification in every program file that uses it.
- Abstract function
- A pure virtual function that does not have a body. Equivalent to an abstract method in Java, but unlike Java, "abstract" is not a C++ keyword.
- Acceptance criteria / acceptance requirements
- A formal, legal document, or a section of a formal, legal document that specifies how a system (software, hardware, or both) will be tested and how it must perform before a client accepts and pays for it.
- Accessor / access function
- A collective term for both getter and setter functions.
- Accumulator
- Accumulators are variables used to build up values over time, often inside loops. The variables must be initialized, usually to 0, before the accumulation process begins. See Accumulators.
- Activation record
- A synonym for a stack frame.
- Actual parameter
- The value in a function call. Another term for an argument. See formal parameter.
- Address
- Each byte of computer memory has a unique location specified by a numeric value in the range [0,n-1], where n is the size of the computer's memory (4-, 8- 16-, and 32-gigabytes are common on current workstations). The numeric value is the byte's address. Most computer data requires multiple bytes of storage, so the address of a data item is the address of the first byte of the data.
- Address of operator
- An operator finds and returns the address of a variable (including instances of structs and classes). If "a" is a variable, then &a is an expression read as "the address of a." See Pointer Operators for illustrations and examples.
- ADT
- An abstract data type.
- Aggregation
- A constructive class relationship that builds large, complex classes out of small, simple ones. Aggregation is typically characterized as a "has a" relationship. For example, "a car has a motor." Each class in the relationship plays a distinct role: car is the whole class and motor is a part class. The relationship operates in only one direction, from the whole to the part. So, it doesn't sound correct to say "a motor has a car." Please see Aggregation: Pointer Member Variables for details.
- aka
- A common abbreviation for "also known as."" Frequently used to introduce synonyms or additional terms.
- AND
- The logical and bitwise AND operators, && and &, respectively, produce a true or 1 value only when both operands are true or 1.
Logical-AND Bitwise AND x y x && y x y x & y F F F 0 0 0 F T F 0 1 0 T F F 1 0 0 T T T 1 1 1 - Anonymous object
- An anonymous object is a fully-constructed and functional object but it doesn't have a name. The compiler automatically creates anonymous objects while evaluating expressions but programmers can also create them when necessary. For example, let Foo be the name of a class with a default constructor and a member function named member:
- Foo* f = new Foo;: creates a new Foo object named f on the heap
- (new Foo)->member();: creates a new unnamed Foo object on the heap; it is an anonymous object because it is not named
- Foo f;: creates a new Foo named f on the stack
- Foo().member();: creates a new unnamed Foo on the stack; it is an anonymous object because it is not named
- ANSI (American National Standards Institute)
- "The American National Standards Institute (ANSI) is a private, non-profit organization that administers and coordinates the U.S. voluntary standards and conformity assessment system. Founded in 1918, the Institute works in close collaboration with stakeholders from industry and government to identify and develop standards- and conformance-based solutions to national and global priorities." An ANSI-compliant C++ compiler must support all the features specified by the ANSI standards, which makes it easier to move program source code between computers that are ANSI-compliant compilers.
- API
- Application Programming Interface. Application programs can provide services to other programs, including access to the data they manage. Programs access the application's services through a set of specifications (an interface) that look like functions and are often described by a list of function signatures. They allow external programs to access the applications' service while "hiding" how the application operates and limiting access to its data.
- Argument
- Strictly speaking, an argument is a value passed from a function call to a parameter in the called function. However, "argument" and "parameter" are often used interchangeable. See Function Arguments and Parameters for details and examples.
- Array
- A fundamental or built-in data structure consisting of an ordered sequence of same-type elements accessed or selected by an index or subscript. An array has one or more dimensions, and each dimension has a size that is fixed when the array is created. Each dimension is zero-indexed, which means that zero is the lowest allowed index value and size-1 is the greatest index value. See Visualizing Arrays for illustrations and examples.
- Arrow operator
- A member selection operator (->). The left hand operand is a pointer to an object (an instance of a struct or class); the right hand operand is a data or function member. See Member Selection.
- ASCII / ASCII code
- American Standard Code for Information Exchange. Sometimes used as a synonym for character or string element. A common or agreed-upon standard for encoding escape and textual characters. The code is typically presented as a table.
- Asciibetical
- A pun created by replacing "alpha" with "ascii" in "alphabetical." It's an insider's joke describing how computers order textual data based on the ASCII code. In ASCII code, upper case letters come before lower case, digits come before letters, and punctuation characters are spread throughout the codes.
- ASCII table
- A listing of ASCII codes. See the following for examples:
- Association
- A constructive class relationship that builds a peer-to-peer connection between two classes. Association is characterized as a "has a" relationship that operates in both directions. For example, it sounds correct to say "a contractor has a project," and it sounds correct to say "a project has a contractor." Please see Association for details.
- Associative data structure / associative container
- A data structure or container whose elements a program accesses by a key value rather than by position (e.g., an array).
- Associative search
- Associative searches apply to data structures containing aggregate data. The aggregate data typically consists of objects instantiated from structures or classes with multiple fields or members. Programs pass a key to associative search algorithms, which search through the data structure for an object with a matching field. The algorithm returns the complete object if it finds a match or an appropriate indicator if it doesn't. For example, a data structure may contain Part objects with a name, a part number, cost, location, and specifications. A search algorithm may search for the name or part number. If the algorithm finds a matching Part, it returns the object to the program to retrieve all the needed Part information.
- Associativity
- The direction, left or right, in which operators with the same precedence are evaluated. For example, A+B+C is evaluated as (A+B)+C. See Order of Operator Evaluation.
- Asynchronous
- Two or more events or actions may occur at different times. An asynchronous function call may return before the called function completes. This behavior is analogous to sending a text message or leaving a voice message - the sender doesn't wait for a reply. See Synchronous.
- Attribute
- In object-oriented analysis, design, and programming, attributes are data that characterize and distinguish between instances (i.e., objects) of the same class. C++ implements attributes as member variables, and Java implements them as instance variables or instance fields. Both languages implement attribute variables in class scope.
- auto
- A keyword that (1) modifies a variable definition, making an automatic variable, or (2) uses type deduction to infer the data type of a variable.
- Automatic variable
- The ALGOL programming language was the first to support automatic variables, and the term generally applies to all languages derived from it. While C++ authors do not universally use the term, in the book introducing C++, Stroustrup (1987, p. 250) states:
There are two declarable storage classes auto and static.
Memory to store the contents of an automatic variable is automatically allocated on the stack when the variable comes into scope and is automatically deallocated when the variable goes out of scope. Languages that support automatic variables have lower overall memory requirements than languages that do not, and they also support recursion. Synonyms are local variables and (less often) stack variables.- Automatic objects are local to each invocation of a block and are discarded upon exit from it.
- Static objects exist and retain their values throughout the execution of the entire program.
Stroustrup, B. (1986, 1987). The C++ Programming Language. Reading, MA: Addison-Wesley.
- Automation
- Automation is memorization through use and practice and has many advantages over rote memorization. Automation ensures that what is memorized is worth the effort, is memorized in context, which helps trigger recall, and is not as quickly forgotten. However, van Merriënboer & Sweller (2005) observe that "automation requires a great deal of practice... . [So,] well-designed instruction should not only encourage schema construction but also schema automation for those aspects of a task that are consistent across problems." (p. 149).
van Merriënboer, J. J. G., & Sweller, J. (2005). Cognitive load theory and complex learning: Recent developments and future directions. Educational Psychology Review, 17(2), 147-177.
B
- BFS
- Breadth first search.
- Big-endian
- Most kinds of computer data are too large to fit into a single byte, so computers store the data as a sequence of bytes. When a programmer defines a variable, the variable name maps to the address of the first byte in the sequence, which is at the lowest or smallest memory address. The computer hardware dictates the byte order of the data in memory. A big-endian computer stores the big end first: it stores the most significant byte (the left-most end) of the data at the lowest location in memory. For example, given the integer 0xaabbccdd, a big-endian computer stores the first or most significant byte, "aa" at the lowest memory address, "bb" next, "cc" after that, and "dd" at the highest address.
- Binary operator
- An operator that requires two operands. For example: a + b.
- Binary tree
- A dynamic data structure that organizes data in memory in a branching, two-dimensional pattern. See Dynamic Data Structures for an illustration.
- Binding
- When an operating system loads a program into main memory for execution, it loads the machine code for each compiled function at some address. We call the process of connecting the function by its address to some program element binding. Two kinds of binding are used in the text:
- Function to call. A function call interrupts the sequence of steps that the computer normally goes through to process each machine code instruction by jumping to the function's address. The program can bind the function call to the function's machine code at two different times: at compile-time, and at run-time.
- Function to object. Member functions are always bound to an object, which is the default target of the function's operations, by the "this" pointer.
- Bit
- A binary digit: a 0 or 1.
- Bitmask
- A pattern of bits (1s and 0s) that is often represented by a symbolic constant. Bitmasks are used with the bitwise operators to set, unset, and to test the setting of bits (often called flags) that control binary configurations. See Bitmasks for illustrations and examples.
- Block
- A block is a delimited sequence of statements. Programming languages delimit blocks in many ways. For example, Pascal delimits blocks with begin and end, and Python uses indentation. C++ and Java delimit each block with an opening and closing brace: {....}. A block is equivalent to a compound statement. Blocks may be nested, and each block defines a distinct scope. Blocks are used to form function bodies, block statements, classes, namespaces, etc. See Blocks for more detail and examples.
- Block statement (compound statement)
- A synonym for a block. Block statements are used wherever a single, simple statement is allowed but where a programmer need to give the computer multiple instructions. Block statements are enclosed in braces but are not terminated with an external semicolon. For example:
Simple Statement Block Statement for (int i = 0; i < 10; i++) cout << i << endl;
for (int i = 0; i < 10; i++) { x = a long calculation; cout << i << << x << endl; }
- Block-structured language
- A computer programming language in which programs consist of a set of blocks.
- BNF
- Originally Backus Normal Form but later changed to Backus-Naur Form. A metalanguage used to define programming languages. A grammar expressed in BNF consists of a set of rules. Each rule has one non-terminal symbol, and one or more possible replacements or substitutions. See Blocks for more detail and examples.
- Bootstrap / bootstrapping / booting
- A bootstrap is a length of material sewed at the top of a boot, aiding the wearer to pull on the boot. Bootstrapping is a self-starting process envisioned as someone grasping the straps of their boots and pulling themselves up. In the context of computing, two uses are relevant:
- The operating system is a program, and in normal operation, it loads and runs application and utility programs. The process of loading and running the OS, the first program, is called "booting."
- In the case of a compiler written in the language it compiles, bootstrapping is the multi-step process of moving or porting the compiler to a different system. For example, the C language compiler is written in C. Developers implement a subset of the C language as a small, simple C compiler in the target system's assembly language. They use the small compiler to compile a more complete version of the language, continuing until the final compiler is fully operational.
- Bottom-up implementation
- A technique for building large, complex programs by starting with the program's small, simple functions. Programmers create simple driver programs that test the functions. See also Top down implementation. See Bottom-Up Implementation for an example.
- Breakpoint
- A breakpoint is a location in a program set by a debugger. When the debugger reaches a breakpoint, it stops execution to allow a programmer to examine parts of the program such as the values saved in variables or calculated by expressions.
- Breadth first search (BFS)
- One of the orders that a program can "visit" or processes the nodes in a tree. Working top to bottom, visits each node on a level before descending to the next (as illustrated by the red arrows). Visiting the nodes in a level requires a queue. Let q be a queue with operations put and get that puts a node at the end of the queue and gets a node from the beginning of the queue respectively. Let root be the top of the tree, the node containing 'A' in the illustration. And let n be a node. The following pseudo code illustrates the BFS algorithm:
q.put(root) while (q.not_empty()) n = q.get() visit(n) for (all sub-trees s of node n) q.put(s)
Although BFS is illustrated with a binary tree, the algorithm works for any non-cyclic tree. The BFS sequence is ABCDEFG. See also Depth first search. - Bridge function
- A function declared as a friend of two or more classes.
- Buffer
- A buffer is something that sits between two or more entities and cushions them as they bump together. A computer buffer is a block of memory that temporarily holds data as the computer moves it from one place to another. A buffer is useful when the data source has a different size or capacity than the destination or when the source and destination operate at different speeds. For example, disk drives provide on-board memory to temporarily hold data as it read from or written to the drive. Computer programs typically create a buffer as an array.
- Buffer overflow / buffer overrun
- A buffer implemented as an array has boundaries (a beginning and end), but it is embedded in and surrounded by memory. If a program tries to put more data into the buffer or array than the array can hold, it overflows or overruns the array's boundaries, overwriting the data stored in adjacent memory. Overflowing a buffer is a well-known software attack. See Arrays And Security and Buffer Overflow Attack.
- Bulletproof code
- Code that reads user input and does not crash or otherwise fail if the input contains data that is not the correct type or in the correct format. The code displays an appropriate diagnostic message and either terminates the program or allows the user to reenter the requested data.
- Byte
- A small unit of digital information, typically consisting of 8-bits. Previously, a byte was synonymous and interchangeable with a character, providing 256 unique characters. Today, most programming languages use or support multi-byte (generally 2-byte) characters, allowing more and larger character sets, which supports more human languages.
- Byte addressable
- Most contemporary computers are said to be byte-addressable, which means that a single byte of main memory is the smallest amount of storage that a computer can address or directly access.
C
- C-String
- C++ programmers have two systems for representing and operating on sequences or strings of characters. C-strings, whose name suggests they were inherited from the older C Programming Language, represent strings of characters as null-terminated character arrays. The null termination character marks the end of the C-string, and any characters following the null terminator are ignored. See the string class for an alternate string representation. See C-Strings for illustrations and examples.
- C-style cast
- An operation inherited from the C Programming Language that converts an expression from one type to another: (new_type)expression;. See Typecast.
- Call stack
- See stack (2).
- Case analysis
- "Case analysis" broadly describes a decision-making process. As used here, it describes an efficient decision-making technique that decomposes a decision problem into a set of situations or cases a program evaluates sequentially. Programmers typically implement cases as if-statements, or sometimes a switch, with an embedded sub-statement. They generally implement sub-statements in loops with either a
break
orcontinue
operator and with areturn
operator in functions. Case analysis orders the cases from the least to most expensive (i.e., shortest to longest running time). This order naturally results in relatively efficient code.Imagine a function that validates the strength of a password saved in the string pw. For the following example, a "strong" password must not be null, must be at least eight characters long, and must contain a digit. Testing a string for null is fast and easy, so the program does that first. Operating on a null string, such as calculating its length or examining its contents, causes a runtime error in many languages, but case 1 ensures that cases 2 and 3 are safe. Getting a string's length is fast, so the program performs that test as case 2. Examining the contents of a string is a longer, linear process, so the program does that test last.
bool password_checker(char* pw) { if (pw == nullptr) // case 1 return false; if (pw length < 8) // case 2 return false; if (pw doesn't contain a digit) // case 3 return false; return true; }
for (file f in list_of_files) { if (f doesn't exist) // case 1 continue; if (f isn't a regular file) // case 2 continue; process f; // case 3 }
- Cast / casting
- See Typecast.
- Categorize
- To place into a category or group. Synonym for classify.
- class
- A keyword that introduces a class specification. A class is an abstract description of a design or programming element that includes data (member variables) and operations (member functions). Cookies and a cookie cutter are common similes used to help understand the relation between classes and objects. The cookie cutter is like a class: it determines the size, shape, and decoration of each cookie, but it is inedible. Cookies are like objects: once the dough is rolled out, any number of edible cookies can be stamped out with the cutter.
Formally, a class name is a type specifier, a programmer-created data type or an ADT. An object is the data created or instantiated from a class. For example, Foo is the name of a class and the variables f1 and f2 are objects:
- Foo f1;
- Foo* f2 = new Foo;
- Class diagram
- UML class diagrams consist of a set of class and class relationship symbols, and describe an object-oriented program's static structure - the time-invariant organization of a program's classes and class relationships. The five UML class relationships are denoted by a set of distinctly decorated lines. Pleas see statechart diagram for a description of dynamic structure.
- Class scope
- An intermediate scope created by defining an identifier inside a class. Class scope lies between local and global scopes: it allows member functions to share data but hides the data from non-member functions.
- Class relationship
- Object-oriented programs consist of a collection of objects that are bound together in such a way that they can cooperate in the solution of a specific problem. The Unified Modeling Language (UML) recognizes five distinct ways that classes and the objects instantiated from them can be connected together. The connections categorize the ways that the classes relate to each other and are called "class relationships." Just as software engineers try to capture some problem detail in each class, they also try to capture some detail with the relationships they choose to connect the classes. See inheritance, association, aggregation, composition, and dependency.
- Class variable
- Class variables are sub-type of member variables. Unlike instance variables that belong to a specific object or class instance, class variables belong to the class as a whole. This observation has several implications:
- A member variable is made a class variable by adding the
static
keyword in the class specification - The memory needed to store class variables is allocated outside any object
- Class variables are defined and initialized in global scope (outside of any class or function), but their scope is restricted by the scope resolution operator
- All objects or instances of a class share a single copy of any class variable
- Outside of class scope, class variables are accessed with
static
getter and setter functions.
foo.h foo.cpp class foo { private: static int count; public: foo() { count++; } static int get_count() { return count; } void big(); };
foo::count = 0; // def & init void foo::big() { . . . }
client.cpp foo f; f.big(); // typical non-static member function call f.get_count(); // works but is not preferred foo::get_count(); // preferred way to call a static function
- A member variable is made a class variable by adding the
- Classify
- To place into a class, set, or group. For example, imagine that we have a bunch of stuffed toys - different kinds and different colors of animals. We could classify them by animal type: all teddy bears in one class, zebras in another class, and squirrels in another. Or, we could classify them by color: all red animals in one class, blue animals in another class, and yellow animals in another.
- CLI
- Command line interface. A character-based mechanism that allows computer users to enter commands as text. A command name is required, but the command may include zero or more options or switches that modify the command, and zero or more command arguments. See also GUI.
- Code review
- A code review is a process where one or more programmers examine code written by a peer. The process's formality can range from informal to formal, and its scrutiny can range from cursory to meticulous. Code reviews may examine any of the following:
- security
- efficiency
- completeness
- clarity
- interface with other project code
- robustness
- adherence to local style
- Cohesion
- Cohesion is an idealistic goal of programs, functions, models, designs, etc. An entity achieves cohesion when no additions improve it but any elimination degrades it. (It has everything it needs and needs everything it has.)
- Collection
- The Java term for a class designed to store and organize objects. For example, an ArrayList or HashMap. Collections are instances of generic classes. Unlike C++ containers, collections can only store objects; fundamental types like int or double must be wrapped in a corresponding wrapper object (e.g., Integer or Double).
- Column-major ordering
- A method of mapping the elements of a multi-dimensional array to the linear address-space of main memory. Elements are ordered by columns from left to right. Less commonly used than row-major ordering. See Supplemental: Row-Major Ordering for an illustration.
- Compile-time
- An operation, action, or event that occurs before or when a program is compiled. There are two important compile-time operations:
- Creating a compile-time constant
- Binding function calls to the function's machine code.
- Compile-time binding
- Function binding that occurs when the compiler system translates a program into machine code and links the resulting object files together. Sometimes called static or early binding. Compare with run-time binding.
- Compile-time constant
- An element whose value is fixed and unchangeable when the compiler component (the second or middle step in the compiler system) translates C++ to machine code. Programmers create compile-time constants in several ways: See Specifying Array Sizes.
- Compiler
- A program that translates a program written in a high-level programming language into executable machine code. The C++ compiler, or more accurately, the compiler system consists of three parts: the preprocessor, the compiler component, and the linker or loader. See C++ Compiler Operation for a detailed description of the C++ compiler.
- Compiler component
- The full C++ compiler system consists of three parts: the preprocessor, the compiler component, and the linker or loader. Both the full system and the middle component are often referred to as "the compiler." When the distinction it's not clear from context, we'll refer to the middle part of the system as "the compiler component."
- Composition
- Composition is a whole-part class relationship. The relationship means "has a" when read from the the whole to the part, and "is part of" when read from the part to the whole. Composition exhibits strong or tight binding. Please see Composition: Embedded Objects for details.
- Compound statement
- A synonym for a block or block statement. See Blocks for more detail and examples.
- Concrete class
- A class that does not have any pure virtual functions. Programs can instantiate or create objects from concrete classes. Compare with abstract classes.
- Concurrent
- Concurrency is the illusion that a computer is simultaneously completing two or more tasks (threads or processes). (Modern computers typically have multiple processing elements or cores, and each one is capable of processing a task independently of the others. But there are always more tasks than processing elements. So, to simplify this discussion, we assume a CPU with a single, unthreaded core.) The computer achieves the illusion by cycling rapidly from one task to the next, briefly running each one. During each cycle, the CPU works on each task until it is complete or it is time to switch to the next task.
- Conditional operator
- The conditional operator, is formed with the ? and : characters, and is the only ternary C++ operator. The operator forms an expression from three required sub-expressions. For example, if v is a variable and exp1, exp2, and exp3 are expressions:
v = exp1 ? exp2 : exp3;
If exp1 evaluates to non-zero, v = exp2; otherwise, v = exp3. See also Conditional Operator. - Console
- The console is a text-based input/output device. Historically, it consisted of a display (a teletype initially and a cathode ray tube or CRT later) and a keyboard. Today, a console still has a keyboard but the display is now a specific window or even a panel inside a window of a larger program. A C++ program accesses the console through a set of objects: cout, cin, cerr, clog, etc.
- const
- A keyword that programmers use to:
- create a symbolic or named constant
- prevent programs from changing values passed to returned from functions
- Constant
- An expression whose value does not and cannot change during program execution. C++ provides several ways of creating constants. See Constants.
- Constructive class relationship
- The UML defines five relationships connecting classes. Software developers consider three relationships, aggregation, composition, and association, as constructive because they bind objects together, forming or constructing complete programs.
- Constructor
- Constructors are specialized object-oriented functions that construct or build an object. They have several features that set them apart from "regular" functions:
- they are called automatically when an object is instantiated
- the function name is the same as their class name
- they do not have a return type (not even void).
- Constructor chaining / constructor delegation
- The process of having one constructor call another constructor. C++ only allows chained constructor calls in an initializer list. Two special cases are worth noting:
- When a subclass constructor calls a superclass constructor, the call must be the first member initializer in the initializer list. See Inheritance With Non-Default Constructors
- When a constructor calls another constructor in the same class, the call is the only member initializer allowed in the initializer list. See Constructor Chaining.
- Container / Container class
- Containers are objects (i.e., instances of container classes) designed to store and organize data. A C++ container can store fundamental types (e.g., int or double) or objects (e.g., instances of structures or classes). The Standard Template Library (STL) provides a standard set of containers implemented as templates. Common examples include See Collection.
- Context sensitive
- Describing a programming element whose meaning depends on the context in which it is used, that is, on its relative position in a program.
- Copy constructor
- A constructor function that copies an existing object. Used to implement pass by value for function arguments. See Copy Constructor.
- CPU
- The Central processing unit or CPU is that part of a computer controlling its operations. Its sub-components read and process the program instructions, read and write data, and perform logical and arithmetic operations.
- Cross compiler
- A compiler running on one system but generating machine code for another. For example, a compiler running on a Linux system but generating code for Windows. Or, a compiler running on an AMD processor but generating code for an Intel processor.
- CRT
- C Runtime library. Often used to describe features, especially functions, C++ inherits from the C programming language.
- Current working directory (CWD)
- The operating system (OS) spawns a process to manage each running program, providing it with a supporting runtime environment. Part of that environment is the process's "location" within the file system, known as its current working directory. The process or program locates needed file system resources (e.g., data files) based on its CWD. Programs can change their CWD while they run; otherwise, their CWD remains as the OS initially provides it.
- Cycle
- Imagine a city map with locations connected by one-way streets. A route or path forms a cycle when the starting and ending locations are the same without traveling in the wrong direction on any street. The map is an analogy for a graph where the locations and streets represent nodes and paths or edges, respectively. Computer scientists use various graphs to represent abstract or intangible concepts. For example, if the graph describes a program, the nodes represent the functions and the edges the function calls, or the nodes can represent objects and the edges of the class relationships connecting them. The states and transitions of State machines are another common example.
The rectangles in the diagrams represent nodes or locations and the arrow's edges or streets.
- An edge or street from location A to B. No cycle present.
- An edge or street from location A back to A. A cycle with length 1.
- Edges from location A to B and from B to A. A simple cycle of length 2.
- Edges from A to B, B to C, C to D, and D to A. A cycle with length 4.
- Multiple edges or streets may enter and exit a node or location, allowing even simple graphs to have many cycles of varying lengths.
D
- Daemon
- A program or process that runs in the background without direct human control. Unlike the newer spelling of "demon" that signifies an evil entity, the older, Greek-derived spelling of "daemon" signifies a neutral entity. See Daemon for more details and an interesting history of how the word was introduced into computing.
- Data member
- A common synonym for a member variable.
- Data structure
- Data structures are containers that store, organize, and retrieve data. They span a wide spectrum from simple arrays to linked structures like lists and trees to externally managed databases. The data can vary in size and complexity from instances of fundamental types to complex objects. Computer scientists often characterize data structures by the operations they support and the relative time taken to complete each operation.
- Data type / type
- A name that summarizes how the bits (1s and 0s) of a single element or "chunk" of information is interpreted and the size (measured in bytes) of that element or chunk. Fundamental data types like int and double are processed directly by the compiler, but programmers can create new data types with class, struct, enum, and typedef. See Data Types.
- Dead code
- Unused source code present in a program. The code may no longer execute, but even if it does, it doesn't affect what the program does. Dead code is undesirable because it "clutters" a program, needlessly increases its size, and may waste execution time and resources. Dead code typically accumulates in a program as it evolves, and old functionality becomes obsolete, for example, a function that the program no longer calls.
- Debugger
- A tool that helps programmers identify and locate bugs in a program. A debugger interprets a program (including those written in a language like C++ that is otherwise compiled). Breakpoints and tracepoints are the two most commonly used debugger features. See Debugging With A Debugger, Loops And The Debugger, and Functions And The Debugger.
- dec
- A manipulator used for reading and writing decimal (base-10) data from and to the console. Decimal output is the default formatting, but dec is useful to reset the format if it was changed by dec or oct. See Manipulators for examples and additional formatting manipulators.
- Declaration
- Programmers give names or identifiers (called symbols) to various program elements like variables, functions, and classes. A declaration is a statement that causes the compiler to enter a name into its symbol table where it records information that it later uses for code generation and program verification. See Declaration.
- Default
- Merriam-Webster, 5(a): "a selection automatically used by a program in the absence of a choice made by the user."
- Default argument
- A value specified as a part of a function's declaration or prototype. When programmers call a function, they may accept the default value or they may override or replace it with an explicit value passed as an argument. See Default Arguments.
- Default constructor
- A constructor function that does not require arguments: it either has no parameters or has default arguments. The constructor uses default values whenever needed to create an object.
- Definition
- A definition is a statement that reserves memory for data or machine code in a program. Sometimes a declaration is also a declaration. See Definition.
- Delegating constructor
- A technique wherein one constructor delegates partial responsibility for initializing an object to another constructor. That is, one constructor calls another constructor. See Constructor chaining.
- delete
- An operator formed by a keyword. delete returns memory allocated on the heap (sometimes called the free store) with the new operator back to the heap so that it can be reused.
- Delimiter
- A character that separates tokens. See Parse.
- Depth first search (DFS)
- One of the orders that a program can "visit" or processes the nodes in a tree. Working left to right, searches as deeply as possible on each sub-tree before processing the next sub-tree. Find the DFS node sequence by beginning with the top node and "walking" the tree counterclockwise (as illustrated by the red arrows). List each node as soon as it's visited. Equivalent to a pre-order traversal. Although DFS is illustrated with a binary tree, it can be used for any non-cyclic tree. The DFS sequence is ABDECFG. See also Breadth first search.
- Dereference operator
- A pointer "refers to" data stored at some memory address. The process of accessing data through a pointer is called "dereferencing" the pointer. For example:
int i = 10; int* ip = &i; cout << *ip << endl;
int* i defines a pointer variable, and the highlighted code dereferences it (in speaking, we say it "dereferences ip"). The statement prints 10. See Dereferencing A Pointer: The Indirection Operator. - Design pattern
- A design pattern is a statement of a common problem and its general, reusable solution. In object-oriented programming, a design pattern describes a set of classes and relationships programmers can easily adapt to a specific design or programming problem.
- Determinate loop
- A loop where the number of iterations are fixed or established before the loop begins running. Although any C++ loop can behave as a determinate loop, for-loops are well-adapted and a common choice for implementing determinate loops. See indeterminate loop.
- DFS
- Depth first search.
- Direct recursion
- A function that calls itself. See Direct Recursion for a simple illustration.
- Dispatch
- A function call.
- Dot operator
- A member selection operator (.). The left hand operand is a non-pointer object (an instance of a struct or class); the right hand operand is a member (data or function). See Member Selection.
- Downcast
- Whenever possible, computer scientists draw inheritance hierarchies or diagrams with the most general class at the top, and with increasingly specific classes below (see the Actor 1 hierarchy). Typecasting creates an expression that changes the class type of one object in the hierarchy into another class type. A cast form a general class high in the hierarchy to a more specific class lower in the hierarchy is therefor a "down cast." See also upcast and Upcasting.
- Dynamic
- A term often used to describe a process or event that takes place while a program is running. See also static.
- Dynamic binding
- A synonym for polymorphism or run-time binding. Also known as late binding or dynamic dispatch. Compare with static binding.
- Dynamic dispatch
- A function call (the dispatch) where the call is bound (see binding(1)) to the function code at runtime (dynamically). A synonym for polymorphism.
E
- Early binding
- Synonym for compile-time binding. Compare with late binding.
- Elaborate
- In general, to elaborate means "to work out carefully or minutely; develop to perfection" (3), or "to add details to; expand" (4). These definitions help us understand what Greeno, Collins, & Resnick (1996) mean when they observe that "Research comparing excellent adult learners with less capable ones confirmed that the most successful learners elaborate what they read and construct explanations for themselves" (p. 19). The observation that "the most successful learners elaborate what they read" holds great promise but is difficult to apply. See elaboration (yellow-highlighted box) for more concrete steps computer science students can take to "elaborate what they read."
Greeno, J. G., Collins, A. M., & Resnick, L. B. (1996). Cognition and learning. In D. C. Berliner & R. C. Calfee (Eds.), Handbook of Educational Psychology (pp. 15-46). New York: MacMillian Library Reference USA.
- Element
- dictionary.com defines an element as "a component or constituent of a whole or one of the parts into which a whole may be resolved by analysis." The individual parts of an array are called elements, and sometimes the parts of a struct or enum are also called elements.
- Encapsulation
- The first of three criterion for establishing an object-oriented system. Encapsulation is the object-oriented concept of combining or packaging data and the functions that operate on that data into an entity called an object. In this sense, encapsulation is synonymous with an object. The second and third object-oriented criterion are inheritance and polymorphism.
- Endianness
- The storage order of multi-byte data. See Big-endian and Little-endian.
- enum
- A keyword for creating an enumeration. Recent ANSI updates have increased the functionality of C++ enumerations, but in their simplest forms, they only way the are used in the text, they are are fast, compact way of creating integer-valued symbolic constants. See Enumerations for more details.
- EOF
- An integer symbolic constant that stands for "end of file." Some functions that read single characters from files return this value to indicate that the read operation has reached the end of the file - the last character has been read. See wc.cpp for an example.
- Epoch
- Dictionary.com defines epoch as "the beginning of a distinctive period in the history of anything." Applied to computer programming, The Epoch is the beginning or zero time on which the computer bases all date calculations. The epoch for Windows computers is January 1, 1980; for Unix, Linux, and macOS computers, the epoch is January 1, 1970.
- Explicit
- Merriam-Webster Dictionary (1(a)): "fully revealed or expressed without vagueness, implication, or ambiguity." dictionary.com (1): "fully and clearly expressed or demonstrated; leaving nothing merely implied." Fully revealed, exposed, or visible. See also implicit.
- Explicit object / explicit argument
- An explicit object or argument is one that is clearly visible in both the function call and the function definition. In the following table, let Foo be the name of a class and x be an instance of Foo, and y be an instance of a class that defines function. Then:
Definition Call Non-member void function(Foo a) {...} function(x); Member void function(Foo a) {...} y.function(x); - Expression
- An expression is a fragment of code that represents a value:
- a constant: 5
- a variable: the current value stored in the variable
- a non-void function call: sqrt(2.0)
- any of the above combined with an operator: a + 5
- Extractor / extractor operator
- The extractor is a binary input operator. The left hand operand is an input stream object (like cin), and the right hand operand is a variable into which the operator sends the input value. The name "extractor" is derived from the operator's behavior: it extracts data from the input stream and stores it in a variable. Programmers create an extractor by overloading the bitwise right-shift operator with a function named operator>>.
F
- Fail-Fast
- A software design strategy favoring aborting or "crashing" a program or system over continuing to run in an anomalous or compromised condition. Compromised programs can waste user time and system resources, corrupt data, and physically damage hardware.
- Feature
- "Feature is a generic word for either an attribute or operation" (p. 26). I also use "feature" as a generic term for member variables and functions.
Rumbaugh, J., Blaha, M., Premerlani, W., Eddy, F., & Lorensen, W. (1991). Object-Oriented Modeling And Design. Englewood Cliffs, NJ: Prentice Hall.
- Field
- One element or variable embedded in an instance of a struct or class. A synonym for member, especially when applied to data.
- Flag
- Flags are variables used to record a program's state or condition. For example, a program might use a flag variable to "remember" on which floor an elevator car is currently located. Programmers often use Boolean (bool) flags when only two states or conditions are possible; otherwise they use integers (int) flags. See Flags for an illustration and example.
- Flow of control
- Imagine printing a program on fan-fold paper and spreading it out on a long table. Place the tip of a marker at the top of main. Mentally run the program while tracing each instruction with the marker as you run it. The marker traces the branches taken by if-statements and coils around the program's loops. You must pick up the marker and move it when the program calls a function, but you return it to the statement following the call when the function ends. The ink trace left on the paper represents the program's flow of control.
- Formal parameter
- A local variable defined in a function. Another name for parameter. See actual parameter.
- Forward declaration
- A forward declaration declares a type or function name before it is defined. Forward declarations are necessary when a compilation unit (a source code or .cpp file) uses a name defined in another compilation unit. When the compiler component encounters a forward declaration it enters the name and any relevant information (e.g., the data type and, in the case of a function, the parameter types) into its symbol table. Function prototypes are a typical example. Forward declarations are necessary to implement association with C++.
- Free store
- Program-managed memory that is available for dynamic allocation. A synonym for the heap.
- friend
- A keyword C++ classes use to allow access to private and protected class features by non-member functions. See friend function.
- Friend function
- Friend functions are not members of the classes that declare them as a friend. Nevertheless, they may access the private and protected features of a class. A function cannot arbitrarily make itself a friend of a class. Instead, a class must declare that a function is a friend by prepending the friend keyword to the function's prototype in the class specification. See friend Functions for details and an example.
- FSM
- Finite state machine.
- Full pathname
- A synonym for absolute pathname.
- Function / function header / function body
- A named and parameterized set of reusable statements that cooperate to perform a task. Data may be passed from arguments in the function call to the function's parameters where statements in the function's body can operate on it. The compiler converts the programming statements in the function to machine code and maps the function's name to an address in memory. A computer runs or executes a function by jumping to the address, running the machine instructions, and then returns to the statement following the function call. Functions have two major components: a header and a body.
The function body consists of the outer most function block and contains the function's statements.
The function header is further divided into a name, a return type, and a parameter list. The header forms the function's interface (how a program accesses and uses it).
- Function binding
- The connection made between a function call and the function's machine code; specifically, when the function call is connected to the function's address in memory.
- Function call
A program is a sequence of statements the computer executes from beginning to end. One kind of statement, a function call, interrupts the sequential statement execution by transferring program control from the call to the function's instructions. In this example, the function arguments a, b, c, and d are evaluated before they are passed to the function parameters x and y. When the function ends or a return operator runs, program control returns to the statement following the call.
- Function prototype
- A function declaration statement. The declaration or prototype must include the function's name, return value type, and the type of each parameter; parameter names are optional. Please see The relationship between a function definition and a prototype.
- Function signature
- In C++, a function's header is synonymous with its signature, and completely specifies its interface. The signature includes the function's name, return type, and parameter types (parameter names are optional). Programmers form function declarations or prototypes by appending a semicolon at the end of a function signature. The signature can also show modifiers like static, and for member functions, it can show the accessibility: public, protected, or private.
- Function scope
- See Local scope.
- Function stub
- A a "dummy" function that contains just enough code that it can compile successfully. Used for Top Down Implementation program implementation.
- Function-style cast
- A notation for converting an expression from one type to another: new_type(expression);. See Typecast.
- Fundamental data type
- A data type that is recognized and processed directly by the compiler (i.e., is not created by a programmer). Also called a primitive data type.
G
- Garbage
- An informal term that describes a random or an otherwise meaningless value.
- Gen-Spec
- A general-specific diagram. Another name for an inheritance hierarchy derived from the meaning of inheritance. Class at the top of the hierarchy or gen-spec diagram denote general kinds of things while subclasses lower lower in the diagram are more specific kinds of things. In the inheritance hierarchy example, a Car is general and a Convertible is a specific kind of Car.
- Generalization
- A synonym for inheritance.
- Getter function
- An access function that allows a client program to access but not change the value stored in a
private
member variable. See also setter function. See Access Functions: Getters and Setters for more detail and examples. - GIGO
- Garbage in, garbage out. If we enter bad data into a program, even if the program is correct, the program will produce bad output.
- Global scope
- A program-wide scope achieved by defining variables and function outside functions and classes.
- Global variable
- Global variables are those that are not defined inside a function or a class. Unless their scope is intentionally restricted to a module created with the static keyword, they can be accessed globally throughout the program. Global variables are a common source of logical errors and should be avoided if possible. Object-oriented programming greatly reduces the need for global variables, but they are still needed in C programs and other specialized situations. See also Local variable and Member variable.
- Golden Age of the World Wide Web
- That period of time between, "Wow, this is really useful!" and "Wow, this is really nonsense!"
-SRW - goto
- A little-used operator that, when combined with a label, forms a goto statement that causes program execution to jump from the goto to the code following the label. The resulting spaghetti code is very error-prone and challenging for programmers to understand and maintain. While there are a few valid uses for goto statements, programmers are generally better served by modern control statements like if, for, while, etc.
- GUI (pronounced "gooey")
- A graphical user interface consisting of a windowed display, keyboard, and pointing device (mouse, touchpad, trackball, etc.). Users control the computer by clicking on icons representing programs or files or selecting items from a menu. See also CLI.
H
- Has a
- A common phrase used to characterize and identify a whole-part class relationship. For example, let Car and Engine be two classes. The statement "a Car has an Engine" makes sense. However, the statement "an Engine has a Car" does not. Therefore, we can conclude that a whole-part relationship connects Car (the whole class) with Engine (the part class). Aggregation and composition are Whole-part relationships that read correctly in only one direction. Association is a more general whole-part relationship that reads well in both directions. Let Project and Contractor represent two classes. Both statements, "a Contractor has a Project" and "a Project has a Contractor," make sense.
- Header / header file
- Header files generally contain declarations such as classes, structures, functions, enumerations, etc. Including header files that only contain declarations multiple times does not cause problems. However, C++ also allows some limited-scope definitions, such as inline functions. Including headers containing definitions more than once will cause a "multiple definition identifier" error. Programmers use #include guard or #pragma once to prevent multiple inclusions. Modules are a new replacement for header files introduced by the ANSI 2020 C++ standard.
- Heap
- A region of memory from which a program can dynamically allocate memory with the new operator. See also stack(2). See Memory Management: Stack and Heap for a more complete discussion.
- Helper function
- Helper functions contain operations shared by multiple member functions. Grouping the common operations in a function and calling it from other member functions eliminates code duplication in the other functions. Programmers also use helpers to manage complexity by decomposing large functions into smaller ones. Although helper functions are class members, programmers excluded them from a class's public interface (i.e., make them unavailable to the application or client programs) by making them
private
. See Using a helper function for an outlined example or fraction.cpp: helper functions for a complete example. - hex
- A manipulator used for reading and writing hexadecimal (base-16) data from and to the console. For example, the statement:
cout << hex << 15 << " " << 16 << endl;
printsf 10
. See Manipulators for examples and additional formatting manipulators. - Hierarchy
- dictionary.com: "any system of persons or things ranked one above another." Cambridge Dictionary: "a system in which people or things are put at various levels or ranks according to their importance." Macmillan Dictionary, 3: "a series of things arranged according to their importance." Merriam-Webster: "a graded or ranked series." Common examples include indented lists and company organization charts. Classes in an object-oriented program can be organized in hierarchies by inheritance and whole-part relationships.
- High-level language
- High-level programming languages are problem-centered. Their features allow programmers to focus on solving a problem rather than on the hardware running the program. C++, C#, and Java are examples of modern high-level programming languages. See also low-level language.
I
- IDE
- An integrated development environment is a program development tool. It is a program providing a single user interface for launching and controlling other development programs: an editor, compiler, dynamic syntax checker, debugger, profiler, etc.
- Identifier
- A name that a programmer gives to or uses to identify a programming element such as a variable, function, or class.
- IEEE floating point standard
- IEEE 754
- The IEEE (Institute of Electrical and Electronics Engineers) 754 standard specifies the internal computer binary format for floating point numbers. Numbers expressed in this format consist of three groups pf bits: an exponent, mantissa, and sign bit. A few bit-patterns do not correspond to "real" numeric values and the standard uses them to represent anomalous conditions: NaN, -NaN (not a number), Inf, and -Inf (infinity).
- Imperative Programming Language
- Programs written in imperative languages consist of a sequence of statements, where each statement is an instruction causing the computer to do one operation. The operations typically cause a state change.
- Implementation inheritance
- An incorrect use of inheritance often used to facilitate message forwarding. A constructive relationship is generally a better choice for connecting the classes.
- Implicit
- Dictionary.com (1): "implied, rather than expressly stated." Cambridge Dictionary: "suggested but not communicated directly." Implied or suggested, but not visible. See also explicit.
- Implicit object / implicit argument
- An implicit object or argument is visible in the function call but not in the function definition. In the following table, let Foo be the name of a class, x be an instance of Foo, and y be an instance of a class that defines function. Then:
Definition Call Non-member void function(Foo a) {...} function(x); Member void function(Foo a) {...} y.function(x); - Include guard
- See #include guard.
- Incremental delivery
- The practice of providing a customer with a partial but useful system as quickly as possible and then incrementally adding features over time.
- Indeterminate loop
- A loop where the number of iterations are not fixed or established before the loop begins running - the number of iterations depends on dynamic conditions evolving with the program execution. Any C++ loop can operate as an indeterminate loop, but while- and do-while-loops are well-suited to the task. See determinate loop.
- Indirect recursion
- A sequence of function calls that form a cycle - a function calls one of its predecessor function before that function returns. See Indirect Recursion for a simple illustration.
- Indirection operator
- Another name for the dereference operator.
- Index
- An integer-valued expression that selects one element of an array; also called a subscript. See Creating and using arrays and Array elements are variables for examples.
- Inf
- An IEEE 754 bit-pattern denoting a calculation resulting in an infinite value. -Inf indicates an infinite value coming from the negative direction.
- Inheritance
- Inheritance, also known as generalization, is one of five class relationships that can exist between two classes. Subclasses inherit all the features of their superclasses (i.e., without redefining them, a subclass acquires all its superclass's attributes and operations). Object-oriented practitioners typically characterize inheritance as an "is-a" relationship. So, we read the accompanying class diagram as "a Sedan is a Car" and "a Car is a vehicle." Several object-oriented features, for example, typecasting, function overriding, and polymorphism, require inheritance. See Inheritance for more detail.
- Inheritance hierarchy
- A hierarchy formed by two or more classes related by inheritance. Subclasses inherit all of their superclass' features For example Sedan inherits all Car and Vehicle features.
- Initializer list
- A comma-separated sequence of initializers introduced by a colon and appearing between a constructor's argument list and body. See:
- Augment list notation for an example of data member initialization
- Calling a superclass default constructor and Constructor call chains for calling superclass constructors with UML inheritance
- Constructing or initializing a part object with a default constructor and Constructing a part object with a parameterized constructor for an example of using initializer lists with UML composition
- Initializing a pointer member with a class constructor for an example of using initializer lists with UML aggregation
- Inline function
- The body of a "normal" function is compiled to machine code once (i.e., there is one copy of the machine code). A call to a normal function transfers control to the function's code, executes the code, and returns to the statement following the call (see Typical function call).
Alternatively, the body of an inline function is compiled to machine code whenever the program calls the function, and the machine code replaces the function call. This process makes inline functions fast but only appropriate for small, simple functions. The
inline
keyword is a suggestion to the compiler and it may be ignored. See Macro vs. inline function (b) for an illustration. - In-order
- One of the orders that a program "visits" or processes the nodes in a binary tree. Let Node be an object with data, left and right pointers, and let visit be an arbitrary operation on node data. The following pseudo code implements in-order processing as a recursive function. Processing begins by calling the function with the top node as an argument.
in-order(Node* n) { if (n->left != nullptr) in-order(n->left); visit(n->data); if (n->right != nullptr) in-order(n->right); }
You can find the in-order node sequence by beginning with the top node, "walking" the tree counterclockwise (as illustrated by the red arrows), and listing the nodes as follows:- left
- list/visit
- right
- Inserter / inserter operator
- The inserter is a binary output operator. The left hand operand is an output stream object (like cout), and the right hand operand is the expression or value that the operator sends to the output stream. The name "inserter" is derived from the operator's behavior: it inserts data into the output stream. Programmers create an inserter by overloading the bitwise left-shift operator with a function named operator<<.
- Instance
- Generally, "a case or occurrence of anything" (dictionary.com). As applied to object-oriented programming, a synonym for an object.
- Instance variable / instance field
- Instance variables are one kind of member variable, but I often use "member variable" and "instance variable" interchangeably because most member variables are instance variables. The memory needed to store an instance variable is allocated inside an object when the object is created or instantiated. In this sense, objects or instances "own" and manage instance variables. See also class variable.
- Instantiate
- The act or process of creating an object or instance from a class or struct.
- Interpreter
- A program that reads a source code file or program one statement at a time without translating the program to any other language. It decodes and executes statement as it reads them. See Running a program written in a purely interpreted language.
- Instruction cycle
- Also known as the fetch-decode-execute cycle. Computers process each machine code instruction in three broad steps:
- Fetch: get the machine code instruction from main memory or cache
- Decode: determine what the instruction means by extracting the op-code and any addresses
- Execute: carry out the instruction
- is a
- A common phrase used to characterize and identify an inheritance relationship. For example, let Car and Sedan be two classes. The statement "a Sedan is a Car" makes sense, but the statements "a Sedan has a Car" or "a Car has a Sedan" do not. Together, these statements suggest that inheritance is the best relationship between a Car and Sedan. Some but not all cars are sedans. This observation suggests that a Sedan is a subclass of Car.
- is part of
- A common phrase used to characterize and identify the aggregation and composition whole-part class relationships. For example, let Car and Engine be two classes. The statement "an Engine is part of a Car" make sense. However, the statement "a Car is part of an Engine" does not. Therefore, we can conclude that a whole-part relationship connects Car (the whole class) with Engine (the part class). Unlike a "has a" relationship that sometimes reads well in both directions, indicating an association relationship, "is a part of" reads well in only one direction, which restricts it to aggregation and composition.
- Iterate
- dictionary.com defines to iterate as "to do (something) over again or repeatedly." Programmers implement iteration in with with loops (for, while, do-while, etc.), which are called iterative statements.
J
K
- Keyword
- In a programming language, a keyword is part of the language's syntax. That means that the word has a special meaning in the language and that its location in a program governed by the language's syntax. That is, the language's compiler or interpreter parses and processes keywords as a part of compiling or running a program. A keyword is also a reserved word.
L
- Late binding
- Synonym for for run-time binding. Compare with early binding.
- Left associative
- An operator that is evaluated left to right. The arithmetic operators (+, -, *, /, and %) are examples of left associative operators. So, if A, B, and C are variables, then A + B + C; is evaluated as (A + B) + C.
- Library
- Software libraries contain general, reusable functions, methods, and classes providing frequently used operations. For example, programmers can use the sqrt library function to calculate the square root of any non-negative value without needing to write their own function. The linker or loader extracts functions from libraries and binds them to application programs. Most programming languages have libraries of common functions and data structures, and large software system like database management systems (DBMS) often include libraries of functions that help application programmers access the system.
- Link
- An instance of the pointers connecting the classes in aggregation and association relationships. That is, when a program instantiates the classes in a pointer-based relationship, it also instantiates the binding connection, which we call a link.
- Linked list
- A dynamic data structure that organizes data in memory in a linear pattern. See Dynamic Data Structures for an illustration.
- Linker
- The name of the third component of the C++ compiler system depends on the hosting operating system. It's called "the linker" on Widows systems, and "the loader" on Unix, Linux, and macOS systems. Regardless of its name, this component joins the object files created by the compiler component to form an executable program.
- Little-endian
- Most kinds of computer data are too large to fit into a single byte, so computers store the data as a sequence of bytes. When a programmer defines a variable, the variable name maps to the address of the first byte in the sequence, which is at the lowest or smallest memory address. The computer hardware dictates the byte order of the data in memory. A little-endian computer stores the littlest end first: it stores the least significant byte (the right-most end) of the data at the lowest location in memory. For example, given the integer 0xaabbccdd, a little-endian computer stores the first or smallest byte, "dd" at the lowest memory address, "cc" next, "dd" after that, and "aa" at the highest address.
- Loader
- The name of the third component of the C++ compiler system depends on the hosting operating system. It's called "the loader" (and implemented by the ld program) on Unix, Linux, and macOS systems, and called "the linker" on Widows systems. Regardless of its name, this component joins the object files created by the compiler component to form an executable program.
- Local scope
- A scope restricted to a function's body or parameter list.
- Local variable
- A variable defined inside a function, including parameters. Local variables have local scope. See global variable and member variable.
- Low-level language
- Low-level programming language are hardware-centered. Low-level language instructions represent a single machine instruction, or, in the case of a macro-instruction, very few machine instructions. The various assembly languages are the most common examples of low-level programming languages. See also high-level language.
- L-value
- A value that can appear on the left-hand side of the assignment operator; an r-value can appear on the right-hand side. See Three characteristics of a variable (c) for a description of how the compiler uses a variable's name to create l- and r-values. Formally, "An l-value refers to an object that persists beyond a single expression" (Wikipedia, downloaded June 7, 2021). L-values are an address in main memory and can be created with reference expressions. See a detailed discussion at Lvalues and Rvalues (C++).
M
- Machine code / machine language
- The lowest-level language the computer "understands." Machine code consists of a sequence of numeric codes, where each code instructs the computer to perform a single operation: ADD, AND, LOAD, STORE, etc. Compilers translate programs written in high-level languages like C++, Java, and C# into machine code. Computers manufactured by different vendors often use different codes for each operation, so machine code is not directly portable between different computers.
- Macro
- A macro definition creates a textual or string mapping: when the macro is expanded, one string of text is replaced by another. C++ creates macros with the #define preprocessor directive For example: #define MAX 100. When the preprocessor expands the macro, it replaces all occurrences of the string "MAX" with the string "100." When used in a program, parameterized macros look like function calls but behave quite differently (see Macro vs. inline function).
- Macro guard
- See #include guard.
- main
- The first function in a C++ program to run (i.e., the function where program execution begins). Although main is not a keyword, we should treat it like it is, and only use it to name the program-start function.
- Manifest constant
- A synonym for symbolic constant.
- Manipulator
- A function specifically designed to work with the
<<
(inserter) and>>
(extractor) operators. C++ organizes the prototypes for manipulators in two header files: The prototypes for parameterless manipulators are in<iostream
, and the prototypes for manipulators with parameters are in<iomanip>
. - metalanguage
- Wikipedia: "In logic and linguistics, a metalanguage is a language used to describe another language, often called the object language.
- Member
- A part of a class or struct. Classes can have both data and function members (see C++ Class Specifications), but structs typically only have data (see Fields/Members).
- Member function
- Member functions belong to or are a member of a class (i.e., they are defined in class scope). Member functions may access all member variables, including those that are private. Please see Member Functions and Program Organization
- Member selection operator
- Programmers use the selection operators, arrow (->) and dot (.), to access the members or fields in class or struct objects. For structures, see Field Selection: The Dot operator and Pointers and The Arrow Operator. For classes, see Figure 3, Creating objects and accessing their features.
- Member variable / member field
- Member variables (aka member fields) are variables declared in a class or structure specification. For example:
class widget { private: int part; double cost; }
struct widget { int part; double cost; }
static
and non-static
. C++ programmers originally used the term "member variable" to describe non-static
variables whose memory is allocated in an object. In contrast, program allocate memory forstatic
variables outside and independent of all objects. Another convention uses the terms instance variable and class variable in place of member variable andstatic
variable. I initially learned and used the original terms; furthermore, instance variables are far more common than class variables. So, throughout the text, I use member variables synonymously with instance variables but specify class variables when using them. - Memory leak
- C++ programs are responsible for maintaining the address of all data allocated on the heap with
new
. A memory leak occurs when the address is lost before the memory is returned to the heap withdelete
. For example, let foo be a user-defined data type:foo* f; ... f = new foo; ... f = new foo;
The second assignment operation overwrites the first address stored in f, causing a memory leak. Leaked memory, informally called garbage, is unusable until the program is restarted. See Memory Leaks for another example. See also Memory leak in Wikipedia. - Message
- Objects in an object-oriented program communicate and cooperate by exchanging or passing messages (i.e., they call each other's member functions).
- Message passing
- An object-oriented term describing how one object can call or dispatch a member function or method defined in another object. For example, if an object has a print member function, a second object can call the print function by sending the first object a "print" message.
- Method
- Like a function, a method is a named and parameterized set of reusable statements that cooperate to perform a task. Methods are typically defined in a class and are bound to an object when called. Although, strictly speaking, methods are equivalent to C++ member functions (as opposed to non-member functions), the terms method and function are often used interchangeably.
- Model-View-Controller
- The Model-View-Controller (MVC) is a design pattern separating a graphical user interface or GUI (pronounced "gooey") into three sub-systems. The View is the visible part displayed on the screen. The Controller provides the human input to control the program. And the Model provides the interface's logical representation; for example, the size and location of a window or button. Users often interact with a program through the visual interface, making it common for a single class to combine the view and controller operations. The Smalltalk programming language introduced the MVC design pattern, and Java bases its swing classes on it.
N
- Named constant
- Synonym for symbolic constant.
- Name collision
- Programmers typically name programming elements based on what they do in a program. A name collision happens when two elements do essentially the same thing but in different contexts or parts of the program, so programmers give them the same name. The problem happens more often when different programmers write different parts of the program. Correcting the collision is relatively easy when one organization creates and owns all the parts. But it's more difficult to fix the problem when different organizations create and own different parts of the code, which is the case when using code extracted from multiple object files in a program.
- namespace
- "namespace" is a C++ keyword that creates a named scope. Formally, "a namespace is a declarative region that provides a scope to the identifiers (the names of types, functions, variables, etc.) inside it. Namespaces are used to organize code into logical groups and to prevent name collisions that can occur especially when your code base includes multiple libraries" (Microsoft Docs). Programs can support multiple functions with the same name if they are declared is different namespaces and their calls are clarified with the namespace name and the scope resolution operator,
::
:namespace dilbert { int foo(); }; namespace wally { int foo(); };
. . . dilbert::foo(); wally::foo(); . . .
- A "using" statement
- Using a fully qualified path consisting of the namespace name, the scope resolution operator, and the element name
#include <iostream> using namespace std; . . cout << "hello world" << endl;
#include <iostream> . . . std::cout << "hello world" << std::endl;
- NaN
- An IEEE 754 bit-pattern denoting a calculation resulting in a value that is not a number. -Nan indicates an infinite value coming from the negative side.
- new
- An operator formed by a keyword that dynamically allocates memory on the heap. Memory allocated with new remains allocated for the program's use until explicitly deallocated with the delete operator.
- Nibble
- 4-bits or half a byte.
- npos
- string::npos is a symbolic constant that represents the longest possible length of a string and is a possible return value of some string functions. Please see The find function.
- nullptr
- A keyword that denotes a special pointer value that indicates that a pointer does NOT point to anything. Although C++ permits programmers to use the older NULL or 0 (the digit 0) to indicate a null pointer, nullptr is preferred. See nullptr for details and examples.
- Null statement
- An empty or missing statement syntactically permitted where a "regular" statement would appear. See Null Statement.
- Null termination character
- A sentinel character marking the end of a C-string. Formed by the ASCII NULL character,
'\0'
(the character zero). - Nuts and bolts
- The phrase "nuts and bolts" suggests that a concept is fundamental and commonplace in a discipline, often reflecting a practical rather than a theoretical perspective.
O
- Object
- Generally, objects are elements or "chunks" of data. They can be a variable, or an instance of a struct or class. More specifically, objects are the fundamental component of the object-oriented paradigm. They organize or encapsulate data together with the operations that are allowed to access the data. Cookies and a cookie cutter are common similes used to help understand the relation between classes and objects. The cookie cutter is like a class: it determines the size, shape, and decoration of each cookie, but it is inedible - it's not the end result. Cookies are like objects: once the dough is rolled out, any number of edible cookies can be stamped out with the cutter.
- Object file
- A complete C++ compiler system includes three components: the preprocessor, the compiler component, and the linker or loader. The compiler component translates preprocessed C++ source code and produces object code. Object code consists primarily of machine code but also contains a dictionary of defined symbols the linker/loader uses to join the object files into a complete program. See Compiler Operation Summary.
- Object-oriented paradigm/model
- Three criterion are necessary to establish the object-oriented paradigm:
- oct
- A manipulator used for reading and writing octal (base-8) data from and to the console. See Manipulators for examples and additional formatting manipulators.
- OMT
- The Object Modeling Technique was originally created by a group working at General Electric, and later was one of three object-oriented software development methodologies "unified" to form the UML. Rumbaugh, J., Blaha, M., Premerlani, W., Eddy, F., & Lorensen, W. (1991). Object-Oriented Modeling and Design. Englewood Cliffs, NJ: Prentice Hall.
- OOA
- Object-oriented analysis.
- OOD
- Object-oriented design.
- OOP
- Object-oriented programming.
- Operating System (OS)
- An operating system is a program that manages a computer's resources, including the central processing unit (CPU), memory, disk drives, etc. Unix, Linux, Windows, and macOS are common operating systems. The computer loads the operating system's kernel when it boots up. Operating systems provide a host environment and various services (e.g., reading and writing files) to running programs.
- Operation
- Merriam-Webster defines an operation as "any of various mathematical or logical processes (such as addition) of deriving one entity from others according to a rule" (5) and "a single step performed by a computer in the execution of a program" (8).
- Operation with assignment
- A shorthand notation that performs an operation and then saves or assigns the result to the left-hand variable. For example, x += y; means x = x + y;.
- Operator
- One or more characters represent an operation or action that a program must perform. Operators operate or work on operands, which are program data items. Operators require one (a unary operator), two (a binary operator), or three (ternary operator) operands. Most operators are formed with one or two non-alphabetic symbols, but a few are denoted with words, for example,
new
,return
, etc. Operators of often used in conjunction with grouping parentheses, but function-call parentheses are not required. - Operator overloading
- The process of or the code implementing an overloaded operator. When overloading operators, programmers must adhere to three rules:
- They may overload most existing operators but may not create new operators
- Overloaded operators have the same associativity and precedence as the existing operators
- Overloaded operators have the same number of operands as the existing operators
- OR
- The logical and bitwise OR operators, || and |, respectively, produce a false or 0 value only when both operands are false or 0.
Logical-OR Bitwise OR x y x || y x y x | y F F F 0 0 0 F T T 0 1 1 T F T 1 0 1 T T T 1 1 1 - OS
- Operating system.
- Overload
- Giving additional or extended meaning to a programming element. For example, C++ overloads the * character, using it to define pointer variables and as the multiplication and dereference operators.
- Overloaded function
- Overloaded functions have the same name and are defined in the same scope. Overloaded functions may have different return types, but they must have unique parameter lists - a different number of parameters or at least one pair of corresponding parameters must be different data types. For example, the y parameters of the two overloaded foobar functions are different, making each parameter list unique:
- foobar(int x, int y)
- foobar(int x, double y)
- Overloaded operator
- A function defined in a class that gives an existing operator a new meaning when operating on one or more instances of that class. The name of the function is operator🙂, but with the operator character or characters replacing 🙂.
- Override
- To replace the current meaning of a programming element with a new meaning or usage.
- Overridden functions
- Overriding functions is a hallmark of the object-oriented paradigm. Overriding functions requires three object-oriented features:
- two or more classes
- inheritance - the classes must be related by inheritance (i.e., be a part of the same inheritance hierarchy)
- overloaded functions must have the same
- name
- return type
- parameter list
P
- Paradigm
- The Free Dictionary defines a paradigm as "a set of assumptions, concepts, values, and practices that constitutes a way of viewing reality for the community that shares them, especially in an intellectual discipline." A software development paradigm describes the fundamental concepts on which the software is designed and implemented. For example, procedural programming decomposes a problem into a set of procedures or functions, and then incrementally implements those functions until the software is complete. Software developed on the object-oriented paradigm identifies objects in the problem domain, abstracts the objects into classes, and implements the software as a set of classes.
- Paradigm shift
- A paradigm shift is "a fundamental change in [the] approach or underlying assumptions" that help us understand a concept or solve a class of problems. For example, Copernicus caused a paradigm shift when he replaced the older geocentric model of the universe with the heliocentric model. Moving from the procedural programming model to the object-oriented model caused a paradigm shift in software development. Paradigm shifts can also happen during software development when the underlying model or language change from one phase to the next.
- Parameter
- Strictly speaking, a parameter is a local variable defined in a function header. Data is passed from an argument in a function call to a parameter in a function body. However, "argument" and "parameter" are often used interchangeably. See Function Arguments and Parameters for details and examples.
- Parameterized macro
- Parameterized macros are an old C language construct for grouping multiple statements (the full name for the construct is macro instruction) so they can be executed with a single name. Although C++ supports parameterized macros with the #define directive, C++ inline functions are more capable and easier to write.
- Parse
- A common computing task where a program analyzes a string one character at a time. Parsing breaks a string into groups of meaningful characters called tokens that are separated by delimiters. For example, many spreadsheet programs export each row of a table as a comma-separated value (CSV) list:
Cranston Snort,(801) 555-1234,115 Elm St.
The exported row has three tokens (name, phone number, and address) separated by two delimiters (the commas). The commands entered in a command-line interface are another common example:gvim driver.cpp fraction.h fraction.cpp
A command-line processor parses the command into a command name (gvim) and three command arguments (driver.cpp, fraction.h, and fraction.cpp). gvim is a text editor and the three arguments are the names of files it will open and edit. The command and argument names are tokens separated by white-space delimiters. - Part
- One of two classes related by aggregation or composition. See Whole / Part Relationships.
- Pass-by-address
- A synonym for pass-by-pointer.
- Pass-by-copy
- A synonym for pass-by-value.
- Pass-by-pointer
C++ passing mechanisms. - 10 to a: by-value (IN)
- x to b: by-pointer (INOUT)
- y to c: by-reference (INOUT)
- Pass-by-reference
- The C++ compiler implements pass-by-reference by mapping two names, the argument name in the function call and the parameter name in the function definition, to a single variable. Arguments must be variables (more accurately l-values). As there is only one variable, changes made to a function parameter also affect the function argument, making pass-by-reference an INOUT passing mechanism. See Pass-By-Reference.
- Pass-by-value
- Pass-by-value (aka pass-by-copy) is C++'s default mechanism for passing data from a function call to the function for all data types except arrays. Any expression may be a function argument. The program evaluates the expression and passes a copy of the resulting value to the corresponding function parameter. Changes made to a function parameter (i.e., the copied data) are discarded when the function ends. See Pass-By-Value.
- Pointer
- A variable that stores or saves an address in main memory. See An abstract representation of a pointer.
- Polymorphism
- One of three characteristics, along with encapsulation and inheritance, of the object-oriented paradigm. It represents a delay in binding a function call to a specific function until the time of the call itself. Common synonyms include "runtime binding," "dynamic binding," "late binding," and "dynamic dispatch," dispatch means "calling a function." Polymorphism is often defined as when sending a message to an object, the object responds appropriately for its type. For example, a program might send the draw message to an object. If the object is an instance of a Circle class, it responds by drawing a circle, but if it's an instance of a Square class, it responds by drawing a square. See Polymorphism Requirements.
- Port
- Moving a program's source code from one platform to another. The new platform may represent different hardware, a different operating system, a different compiler, or any combination of the three.
- Portable data type
- A descriptive but informal synonym for type alias. Whereas a type alias is created with a typedef statement, a portable data can also be created with a #define.
- Portable
- Describing program source code that is easily moved between platforms. See Port.
- POSIX
- Portable Operating System Interface. "POSIX... is a set of standard operating system interfaces based on the Unix operating system. The need for Standardization arose because enterprises using computers wanted to be able to develop programs that could be moved among different manufacturer's computer systems without having to be recoded. Unix was selected as the basis for a standard system interface partly because it was 'manufacturer-neutral.'' However, several major versions of Unix existed so there was a need to develop a common denominator system." WhatIs.com. Unix, Linux, and macOS are mostly POSIX compliant.
- Post-order
- One of the orders that a program "visits" or processes the nodes in a binary tree. Let Node be an object with data, left and right pointers, and let visit be an arbitrary operation on node data. The following pseudo code implements post-order processing as a recursive function. Processing begins by calling the function with the top node as an argument.
post-order(Node* n) { if (n->left != nullptr) in-order(n->left); if (n->right != nullptr) in-order(n->right); visit(n->data); }
You can find the post-order node sequence by beginning with the top node, "walking" the tree counterclockwise (as illustrated by the red arrows), and listing the nodes as follows:- left
- right
- list/visit
- Precedence
- The priority assigned by a programming language to an operator, and used to determine, in part, the order in which operations are evaluated. Expressions created with high-precedence operators are evaluated before expressions with lower-precedence operators. For example, in the expression a+b*c, the sub-expression b*c is evaluated first. See Order of Operator Evaluation.
- Pre-order
- One of the orders that a program "visits" or processes the nodes in a binary tree. Let Node be an object with data, left and right pointers, and let visit be an arbitrary operation on node data. The following pseudo code implements pre-order processing as a recursive function. Processing begins by calling the function with the top node as an argument.
pre-order(Node* n) { visit(n->data); if (n->left != nullptr) in-order(n->left); if (n->right != nullptr) in-order(n->right); }
You can find the pre-order node sequence by beginning with the top node, "walking" the tree counterclockwise (as illustrated by the red arrows), and listing the nodes as follows:- list/visit
- left
- right
- Preprocessor
- The full C++ compiler system consists of three parts: the preprocessor, the compiler component, and the linker or loader. The preprocessor handles directives that begin with the # character (e.g., #include or #define) appearing in C++ source code files. It writes the processed source code to a temporary file, which the compiler component processes and deletes.
- Primitive data type
- A data type that is recognized and processed directly by the compiler (i.e., is not created by a programmer). Also called a fundamental data type.
- private
- A keyword modifier that limits access to class members to instances of the same class. Note that the affect of private is at the class level rather than at the object level. For example, class alpha has a private data member named omega. One instance of alpha may access the omega member of another alpha object with a member function:
class alpha { private: int omega; public: alpha(int o) { omega = o; } alpha add(alpha a) { return alpha(alpha + a.omega); } };
See Feature Visibility. - Process
-
A process is an instance of a running program. Most modern operating system launch or run a program by loading the program's executable code into main memory and spawning a data structure called a process to manage it. Each process has a unique, non-negative identification, called a process ID or pid. The process stores the location of the executable code, the current program counter, any open files, and any other resources the process uses. Each program instance (i.e., each time the OS loads a program into memory and runs it) is a separate process; so the same program may be running simultaneously as many distinct processes.
- «process»
- A standard UML stereotype (see The UML Class Symbol) labeling a class's process functions - functions that perform general, algorithmically "interesting" operations on some or all of an object's member variables. Process functions typically represent a significant part of a class's public interface.
- protected
- A keyword modifier that limits access to class members to instances of the same class and its subclasses. For example, class beta is a subclass of alpha and may access its protected members:
class alpha { protected: int omega; public: alpha(int o) { omega = o; } alpha add(alpha a) { return alpha(alpha + a.omega); } }; class beta : public alpha { void display() { cout << omega; } };
See Feature Visibility. - Prototype
- Short for function prototype.
- Pseudo Random Number Generator (PRNG)
- A function or class creating a sequence of numbers that "seem" random (they pass some statistical tests of randomness) but are functionally related and predictable. The sequence forms a long cycle, and programs often use relatively short sub-sequences as random numbers. To improve the appearance of randomness, a seed determines where in the cycle the sub-sequence starts.
- public
- A keyword modifier that permits access to class members by all objects and functions. See Feature Visibility.
- Public interface
- A class's public interface are its non-
private
features - generally itspublic
features but occasionally includingprotected
ones as well. Variables are typicallyprivate
, so client code uses objects through the class'spublic
functions. Classes provide limited access to theirprivate
variables through getter and setter functions when there is a valid reason to do so. Please see A Class's Public Interface. - Pure virtual function
- An abstract function that does not have a body; the pure specifier,
= 0
(the digit zero), replaces the body. For example, imagine a class named Shape has a draw function. A shape is too ambiguous or abstract to draw, so we make the function a pure virtual function:virtual void draw() = 0;
See abstract class for details. See concrete class and virtual function.
Q
- Quicksort / Quicksort Algorithm
- Quicksort is a divide-and-conquer algorithm that partitions data around a pivot point into two sub-sets. It's an efficient algorithm that generally provides O(n log n) performance, where n is the number of elements in the initial set. Its efficiency makes quicksort a common choice for inclusion in language APIs or libraries. Java, for example, uses it for most of the Arrays.sort and Collections.sort methods. C and C++ include qsort in their libraries (see Library Functions: qsort And bsearch). In rare cases, for example when the original data is already sorted in reverse order, quicksort exhibits poor performance: O(n2).
R
- R-value
- A value that can appear on the right-hand side of the assignment operator; an l-value can appear on the left-hand side. See Three characteristics of a variable (c) for a description of how the compiler uses a variable's name to create l- and r-values. Formally, "An r-value is a temporary value that does not persist beyond the expression that uses it" (Wikipedia, retrieved June 7, 2021). See a detailed discussion at Lvalues and Rvalues (C++). Programmers also use r-values in more places than just assignment statements. Some examples of r-values (highlighted):
- c = sqrt(pow(a,2) + pow(b,2));
- cout << sqrt(pow(a,2) + pow(b,2)) << endl;
- Radix
- "Radix" is the number of unique digits in positional numbering system and is a synonym for "base." For example, decimal or base-10 has ten unique digits, 1-9, binary or base-2, has two digits, 1 and 2, and hexadecimal has 16 digits, 0-9 and a-f. In a positional numbering system, a digit's position within a number represents a multiplying value. For example:
- 12310 = 1×102 + 2×101 + 3×100
- 12316 = 1×162 + 2×161 + 3×160
- Random Number Generator (RNG)
- Usually short for pseudo random number generator or PRNG
- Record
- A collection of related data. For example, a student record might contain the student's name, identification number, local and permanent addresses, home and mobile phone numbers, GPA, etc. C++ programs can represent records as structures or classes.
- Recursion
- Recursion occurs when a sequence of function calls executes a function while it continues running from a previous call in the sequence.
- Direct Recursion: A function calls itself
- Indirect Recursion: takes place during a sequence of function calls when one function in the sequence calls another function in the sequence before the functions in the sequence terminate
- Reentrant
- Reentrant code allows multiple, simultaneous function invocations or instantiations - meaning that it is safe to call a function while it's still running. Recursion is a special case of reentrancy where a function calls itself either directly or indirectly. More general reentrancy also supports concurrent access by other threads or processes. Reentrant functions cannot use
static
or global variables. - Relative pathname
Most general-purpose operating systems implement a hierarchical or tree-like file system with its root directory or folder at the top. A relative pathname consists of a sequence of zero or more directories followed by a file or directory name. The path begins with the current working directory (CWD) and proceeds to the file or directory named at the end. A path separator character, "\" for Windows systems and "/" for Unix, Linux, and macOS systems, separates each path element (directory or file name). Relative pathnames begin in one of three ways: with the name of the target file or directory, "." representing the current directory, or ".." representing the parent directory. The illustration shades the CWD, matching the text color in the examples:
- file
- file2
- ./file2
- .\file2
- ../../file3
- ..\..\file3
- Requirements / requirements document
- A formal list of features and behaviors (requirements) that a program must satisfy. The requirements are often specified in a legal contract called a requirements document. A requirements document may include a section called acceptance criteria that details how the client will verify that the software meets the stated requirements. The document may also specify any penalties if the software fails to satisfy the requirements by the agreed time.
- Reserved word
- Programmers cannot use a reserved word as an identifier in a program. Generally, a reserved word is also keyword, but there are exceptions. For example, in Java, delete is reserved but is not a keyword, thus preventing programmers from using it in a Java program.
- Resource
- Anything that a program, process, or thread needs to complete its assigned tasks. A resource may include data or hardware.
- return
- An operator that terminates a function and returns control to the next operation following the function call. Non-void functions (functions whose return-type is not void) must have a return a statement to send the result of the function back to the caller. See Function Return, Part 1 for illustrations and examples.
- Right associative
- An operator that is evaluated right to left. The assignment operator is the most common example of a right associative operator. For example, if A, B, and C are variables, then A = B = C; is evaluated as A = (B = C).
- Rote memorization
- Memorization that occurs through repeated rehearsal, or "the use of memory... with little intelligence" (Merriam-Webster). It is appropriate for information with little underlying structure or semantic content. For example, the color codes used to specify the resistance values of resistors. The colors are arbitrary but a user must know them to understand a circuit's behavior. Rote memorization is often a starting point for learning, but is rarely the end. See automation.
- Row-major ordering
- A method of mapping the elements of a multi-dimensional array to the linear address-space of main memory. Elements are ordered by rows from top to bottom. More commonly used than column-major ordering. See Supplemental: Row-Major Ordering for an illustration.
- Rule of thumb
- A rule of thumb is "a general or approximate principle, procedure, or rule based on experience or practice, as opposed to a specific, scientific calculation or estimate."
- Run-time
- An operation, action, or event that occurs while a program is executing or running. See Compile-time
- Run-time binding
- Function binding that takes place at the time of the function call. A synonym for polymorphism. Also called late or dynamic binding, or dynamic dispatch. Compare with compile-time binding.
- Runtime stack
- See stack (2).
S
- Scenario
- "A sequence of events especially when imagined" Merriam-Webster. Software designers and testers frequently describe a program's behavior as a set of scenarios, each depicting a program's response to a specific set of conditions. User input or other external events typically trigger each scenario, which is a snapshot of the program's behavior as it responds to the triggering event.
- Scope
- The location in a program where an identifier is visible and accessible. C++ provides three named scopes: local, global, and class.
- Scope resolution operator
- It is illegal to define two or more programming elements with the same name within the same scope. But if the names are resolved or clarified, it is okay to use or access multiple elements with the same name in the same scope. The scope resolution operator,
::
, resolves the scope of conflicting names. C++ uses it primarily as a binary operator to complete a variety of object-oriented tasks and less frequently as a unary operator to distinguish between local and global scope.- Binary operator: The operator ties a programming element to its declaring scope. The left-hand operand is a scoping construct (a class or namespace) name, and the right-hand operand is a member name. C++ requires the operator to:
- define member functions outside of a class
- create a class-specific symbolic constant
- access namespace functions without the uses keyword
- chain overridden member function calls. The next chapter illustrates how to override and chain constructor and general member function calls.
- Unary operator: the right-hand operand is the name of a global variable. Used to distinguish between global and local variables when there is a name conflict:
int counter = 10; // global definition void function() { int counter = 5; // local definition cout << counter << endl; // accesses local variable cout << ::counter << endl; // accesses global variable }
The code fragment prints 5 and 10.
- Binary operator: The operator ties a programming element to its declaring scope. The left-hand operand is a scoping construct (a class or namespace) name, and the right-hand operand is a member name. C++ requires the operator to:
- Seed
- A pseudo random number generator creates a long cycle of random-like numbers. Programs typically use relatively short sub-sequences of the longer cycle. The seed is a numeric value that determines where the sub-sequence begins (i.e., the first sub-sequence value). Only one seed operation is necessary for each sub-sequence. To reduce the chances of the generator returning the same sub-sequence at different times, the seed is often based on a clock. If the seed operations are separated by time greater than the clock update time (i.e., a clock "tick"), the sub-sequences are different.
- Selection operator
- See Member selection operator.
- Sending a message
- An object-oriented term that means to call one of an object's non-private functions.
- Sentinel
- A special, non-data value stored in a data structure to mark a boundary, such as the start or end of data.
- Setter function
- An access function that allows a client program to change the value saved in a
private
member variable while permitting the class designer to maintain a degree of control. Getter functions can validate data before allowing a change, for example, disallowing a negative length. They can also validate or modify the data's format before saving the new data; for example, a setter could change "Jan 25, 2025" to "01/25/2025" before saving. See also getter function. See Access Functions: Getters and Setters for more detail and examples. - Sequence diagram
- UML Sequence diagrams visually represent the sequence of messages (function calls) objects in an object-oriented program exchange. There are variations between UML versions and authors, and the illustration omits many diagram features here, but the adjacent figure illustrates the most common and useful. The arrows can point
either left or right to denote the direction of the message or response. Time increases from top to bottom, so the arrows' horizontal position denotes their relative calling sequence.
- The horizontal rectangles at the top represent objects and show their names and class types.
- The vertical dashed lines are the objects' lifelines denoting the time during program execution when the object exists.
- The vertical rectangles are the objects' activation boxes, showing when the object executes one of its functions.
- The solid or filled arrowheads represent function calls.
- The dashed arrows are function returns.
- The open-head arrows are asynchronous function calls.
- Short circuit evaluation
- A situation where the result of a logical expression is determined without completely evaluating the expression. For example, if En is Boolean-valued sub-expression, then:
- Given E1 && E2, if E1 is false, then the expression is false, and E2 is not evaluated
- Given E3 || E4, if E3 is true, then the expression is true, and E4 is not evaluated
- Side effect
- A function operation that does not affect the function's return value. For example, printing output.
- Signature
- See Function signature
- size_t
- A type alias or portable data type for an unsigned integer data type appropriate for representing the size of a programming structure. Type aliases are typically created with a typedef statement:
typedef size_t unsigned int;
- Slicing
- When done with a automatic or local variables, C++ implements an upcast operation by copying a subclass object to a superclass. However, the superclass object does not have enough space to store the entire subclass objects, so the subclass portion is "sliced off." See Slicing for an illustration.
- Source code
- A sequence of human-readable statements or instructions adhering to the syntax of a programming language (e.g., C++, Java, C#, Python, assembler, etc.), that express a solution to a given problem. See High-level vs. low-level programming languages and Machine Code.
- Spaghetti code
- techopedia: "Spaghetti code is a slang term used to refer to a tangled web of programming source code where control within a program jumps all over the place and is difficult to follow. Spaghetti code normally has a lot of GOTO statements and is common in old programs, which used such statements extensively."
- Specification
- Applied to a software system, a specification is a formal statement of one system requirement. A set of system requirements may be assembled into a requirements document to create a formal contract between a client and a software developer.
- Stack
-
- A general-purpose data structure. A stack must support at least two operations: push and pop. Data is "pushed onto a stack" and is later "popped off the stack." Programmers often describe stacks as a last-in, first-out (LIFO) data structure, which means that the last data item placed on the stack is the first data item removed. See Demonstrations of stack behavior for an illustration.
- The runtime stack, the call stack, or simply "the stack." A region of memory where programs store local variables, expression values, and function return information. We call this region of memory "the stack" because we treat it as such, and it behaves as such: we remove stored data from the stack in the opposite order we enter it. Recursive function calls and stack frames illustrates how programs manage function calls on the call stack. See also heap. See Memory Management: Stack and Heap for a more detail.
- Stack frame
- A data structure pushed on the stack (2) whenever a function is called (also called an activation record). The function's local variables, including its parameters, are stored in the stack frame along with the return address - the address where execution resumes when the function ends. See The relationship between a function call and a stack frame.
- Stack variable
- A less common synonym for local variable or automatic variable.
- State
- "State" is used to describe individual objects, programs, and systems consisting of programs, hardware, or both. Three related usages are in common use:
- Together, the current values of all program or object data defines the program's or object's state.
- The current condition or activity of a program or object. Simple programs or objects might only have two states, which we can represent with a Boolean variable. For example, a stopwatch may be stopped or running. More complex programs or objects may have many states, which we can represent with integers. For example, an elevator car may stop at any floor either going up or down.
- A graphic symbol appearing in a state machine diagram that represents one of a finite number of states of a system. State-symbols are often illustrated as circles, rectangles, or "round-tangles" (rectangle with rounded corners).
- Statechart diagram
- A UML diagram that shows a state machine describing an object's dynamic behavior - how is changes with time. Not all objects in an object-oriented program have dynamic behavior and only those that do have associated statechart diagrams. Please see Class diagrams for a description of static structure.
- State machine
- A state machine (aka an automation) is an abstract model used to design and describe algorithms, electronic devices, and complex software systems. Applied to electronics and software, state machines are finite and deterministic. A finite state machine (FSM) consists of a finite number of states, transitions, and inputs. A deterministic state machine may has at most one transition out of a state for a given input.
Rectangles with rounded corners represents states. Arrows denote transitions, which are valid ways that a machine can change from one state to another. Transitions are typically labeled with an input and an action. Input can be textual data entered by a user or read from another source, or it may be an event (e.g., a button press, a temperature or pressure reaching a threshold, etc.). An action may be a function call or a physical response (e.g., a fan starts or a valve opens). The forward slash character is part of and introduces the action. The solid disk denotes the initial or start state, the state in which the machine begins running. A solid disk in the center of a circle denotes a halt or accept state - a state in which machine may stop running. If the machine is attempting to match input to a pattern, entering an accept state indicates that the input matches the pattern and the input is accepted. - Statement
- A statement is a single instruction that tells a computer to do one task. See Statement for more details and examples. See also block statement.
- static
- A modifier that changes the behavior of local variables and class members.
- Local variables: The memory in which static local variables are stored is allocated when a program is first loaded into memory to run and remains allocated until the program terminates. The static keyword does not change a variable's scope, but it allows the variable to retain its contents between function calls. See Storage Modifiers: The auto and static Keywords for more detail.
- Member variables and functions. Non-static members belong to or are bound to instances of a class (i.e., to objects). static members belong to the class as a whole - they are not bound to an object - and are called class variables or class functions. C++ implements class variables and functions with the static keyword. Class (static) functions to not have a "this" pointer and may not access non-static members. See static Data And Functions.
- Static binding
- Synonym for compile-time binding. Compare with dynamic binding.
- Stereotype
- Optional labels appearing in UML diagrams. The UML encloses stereotypes in matching guillemet characters: « and ». The UML includes some standard stereotypes (e.g., «constructor», «process», and «helper») or class designers can create new ones as needed.
- string
- The name of a C++ API class that provides a feature-rich implementation of the abstract string type. See The C++ string Class.
- String
- An abstract data type or ADT consisting of a sequence of characters, and implemented in C++ programs as C-strings or instances of the string class.
- String literal
- A string constant formed by enclosing zero or more characters within double quotation marks. C++ uses character pointers (char*) to represent and manipulate string literals. See Constants.
- struct / Structure
- Programmers use the struct keyword to specify or create a structured or aggregate data type. An aggregate data types consists of multiple data elements called fields or members that are accessed by name with the dot or arrow operator. By default, structure members are public while class members are private. See Structures for details and illustrations.
- Stub / stub function
- A "dummy" function that contains just enough code that it can compile successfully. Used for Top Down Implementation program implementation.
- Subscript
- Given the tight relationship between the matrices and vectors used in mathematics and C++ arrays, subscript is a common synonym for index.
- Substitutability
- The ability to substitute or replace an instance of a superclass with an instance of a subclass. Upcasting is based on the substitutability principle. See Substitutability for an example.
- Symbol
- The smallest unit of meaning in a program. Symbols consist of one or more printable characters. Common symbols include operators, keywords, and identifiers.
- Symbol table
- A data structure that stores information about programmer-named components: variables, functions, structures, classes, etc. Basic information includes the component's name, scope, and type. Information for functions includes the number and type of the parameters. Class information includes the number and type of the class's members. If the class is a subclass in an inheritance relationship, the symbol table also includes its superclass. See An abstract symbol table (2) for an example.
- Symbolic constant
- Giving a name to a value that does not change while the program runs. See Symbolic, Named, or Manifest Constants.
- Synchronous
- Two or more events or actions coincide. A synchronous function call requires the caller to wait until the called function runs to completion. This behavior is analogous to making a phone call - the caller must wait until the called party answers. See Asynchronous.
- System call
- A system call is a "programmatic way in which a computer program requests a service from the kernel of the operating system on which it is executed." Operating systems manage a computer system's resources, sharing them among the many running programs. System calls form the operating system's API through which programs can access system resources. For example, accessing a file on a drive or displaying text on a screen or in a window. On older systems, programs placed an op-code (i.e., an operation code) in a hardware register and generated a trap or software interrupt. System calls look more like "regular" functions on modern operating systems.
T
- Template
- A C++ construct that allows programmers to generalize the data type of function parameters and class member variables. Programmers implement templates in two ways:
template <class T>
template <typename T>
- Ternary operator
- An operator that requires three operands. The the conditional operator is the only ternary operator in C++ and Java.
- this
- When a program calls a member function, it passes the address of the object bound to the function as an argument. The function stores the object's address in the pre-defined "this" pointer parameter. See the illustration at Visualizing The "this" Pointer. The keyword this allows a member function to access the bound or calling object when necessary. For example, the this pointer is used to distinguish between constructor and setter parameters and class-scope variables with the same name or where a member function must explicitly refer to the bound object (see for example Overloaded assignment operator).
- This object
- A synonym for an implicit object.
- Thread
- A thread, short for thread of control, is the concurrent execution of a function. Imagine that we print a computer program on fanfold paper and spread it out on a long table. We dip our finger in ink and place it at the beginning of "main." As we mentally execute the program, we allow our finger to move from one statement to the next, tracing the statements as we run them. The ink trail our finger leaves is the "thread of control." Whereas processes are instance of programs, threads arise from functions, so a process can have many threads.
- Token
- A group of characters that together has a specific meaning or use. See Parse.
- Top down implementation
- A technique for building large, complex programs by starting with the program's starting point (i.e., with main). Programmers test the high-level logic by "stubbing in" the functions needed to complete the logic. A function stub is a "dummy" function that contains just enough code that it can compile successfully. See also Bottom up implementation. See Top Down Implementation for examples.
- Tracepoint
- A tracepoint is a location in a program set by a debugger that includes a programmer-specified action. When the debugger reaches a tracepoint it carries out the action, which can be as simple as displaying output indicating that the tracepoint was reached, or evaluating a complex expression.
- Truncate
- Shorten or cutoff. Applied to computer data, it means to discard any fractional value. When truncated, 2.999999999 becomes 2, without any fraction amount. Please note that truncation is not the same as rounding.
- Truncation error
- A loss-of-precision error caused by an incorrect truncation. For example, a program uses two integer variables to calculate an average:
int sum = 0; int count = 0; while (there is data) { count++; sum += data; } double average = sum / count;
As sum and count are integers, the division operation is evaluated with integer arithmetic, which truncates or discards any fractional amount before saving the result in average. - Type
- See Data type.
- Type alias
- A "dummy" placeholder type-name. A type alias is a synonym for an existing data type and programmers may use the alias and exiting type interchangeably. Alias names are typically shorter or convey more information than the exiting type name. Programmers create a type alias with a typedef statement, for example:
typedef size_t unsigned int;
Ortypedef size_t unsigned long;
The compiler will replace the alias with the "real" type most appropriate for a given system (hardware and operating system). - Typecast
- A cast, a typecast, or casting converts a value from one data type to another. The beginning and ending data types must be similar. For example, it's possible to cast a double to an int because they are similar in the sense that both are numeric types, but it is not possible to cast a string to an int because they are too dissimilar. It is only possible to cast objects from one class-type to another if the two class-types are related by inheritance. Two simple forms of casting are in common use:
- (new_type)expression // C-style cast
- new_type(expression) // function-style cast
- Type deduction
- A process that allows the compiler to deduce or infer the data type of a variable based on information presented elsewhere in the program. For example, given the definition int counter;, the compiler can deduce the type of max and min as int when they are defined:
auto max = counter;
decltype(counter) min;
- Type promotion
- An automatic type conversion or typecast from a narrow type to a wider type. "Narrow" and "wide" refer to the range of storable values and not to the number of bytes allocated to store the data. For example, on a 32-bit computer, a long and a float are both 4-bytes, but a float can store values in the range ±3.4×10±38 versus about ±2 billion for a long. So, float is wider than long. See Data Types and Sizes.
- Type specifier
- The name of a data type. The name may be one of the fundamental, built-in types such as int, double, or char (see C++ fundamental, built-in, primitive data types). Alternatively, the name may be a programmer-created type specified with a struct, enum, class, or typedef.
- typedef
- An operator that creates a new (usually shorter) name for an existing data type. typedef statements typically combine multiple names or operators to form new, more compact names. The new name may not contain spaces, but the existing type, between typedef and the new name, may contain spaces. For example:
typedef unsigned long ulong;
typedef struct _file { ... } FILE;
(Creating the C programming language file pointer. The FILE type is opaque, so "_file" and the structure field names and types are invisible and may vary between systems.)
U
- UML
- See the Unified Modeling Language.
- Unary operator
- An operator that requires one operand. Most operands follow the operator: -N or &counter. A few unary operators follow their operand: counter++.
- Unified Modeling Language
- "The Unified Modeling Language (UML) is a graphical language for visualizing, specifying, constructing, and documenting the artifacts of a software-intensive system" (Booch, G., Rumbaugh, J., & Jacobson, I. The Unified Modeling Language User Guide (2nd ed.). Addison-Wesley, Upper Saddle River, NJ. 2004, p. xiii). It combines and unifies the three previous design languages created by the three authors.
- Unreachable code
- Inaccessible source code, code that a program can never execute. For example:
void function() { ...; return; ...; // unreachable code }
loop { part 1; break or continue; part 2; // unreachable code }
- Upcast
- Typecasting creates an expression that changes the class type of one object in the hierarchy into another class type. Answering the question, "why 'upcasting'?," Eckel (1995) states "The reason for the term is historical and is based on the way class inheritance diagrams have traditionally been drawn: with the root at the top of the page, growing downward... . Casting from derived to base moves up on the inheritance diagram, so it's commonly referred to as upcasting" (p. 526). He further states that "You can also perform the reverse of upcasting, called downcasting. See also Upcasting.
Eckel, B., (1995). Thinking in C++. Englewood Cliffs, NJ: Prentice Hall.
V
- Variable
- A variable is a named location in main memory. A variable definition requires a name and a data type. The size of the variable, measured in bytes, is determined by the data type. See Variables for an illustration and details.
- Virtual function
- The C++
virtual
keyword enables a function to operate polymorphically. See Requirements For Polymorphism for a complete list. - Virtual machine
- A computer program that emulates a computer system (either hardware, an operating system, or both) by interpreting individual machine code instructions or system calls.
- Virtual memory
- If a program's demand for main or primary memory (RAM) exceeds what is available, most general-purpose operating systems substitute secondary memory or storage (e.g., a disk drive) for it. Computer scientists call this technique virtual memory. It greatly expands a program's effective memory at the expense of running much slower.
- VM
- A virtual machine.
- Vtable
- A table the compiler creates for classes with one or more
virtual
functions. The table contains an entry for eachvirtual
function in a class. See Implementing Polymorphism for illustrations and examples. - V-pointer / vptr
- A virtual pointer the compiler adds to each object instantiated from a class with one or more virtual functions. Constructors initialize the vpter to point to a class's
virtual
functions, and all polymorphic function calls are made indirectly through the pointer. See Implementing Polymorphism for illustrations and examples.
W
- Width
- A term describing the relative dynamic range of values a specific data type can represent. For example, the range of a four-byte
int
is -2,147,483,648 to 2,147,483,647, and the range of a four-bytefloat
is -3.4028234×1038 to 3.4028234×1038. So, while afloat
has fewer digits of precision than anint
, it is nevertheless wider. - Whole
- One of two classes related by aggregation or composition. See Whole / Part Relationships.
- Whole-part
- The whole-part class relationship is a technique for building large composite or whole classes from smaller component or part classes. Aggregation and composition build different kinds of whole-part connections.
- Working definition
- An explanation of a term used for a specific or special purpose. Wiktionary: "A definition that is chosen for an occasion and may not fully conform with established or authoritative definitions."
X
- XOR
- The exclusive-OR operator, ^, is a bitwise operator (without a corresponding logical operation) that is true or 1 only when the operands are different.
x y x ^ y x y x ^ y F F F 0 0 0 F T T 0 1 1 T F T 1 0 1 T T F 1 1 0 - xyzzy
- The "Colossal Cave Adventure" (aka "advent" because program names were initially limited to six characters) was one of the earliest computer games. I played the game in the late 1970s on a Digital Equipment Corporation (DEC) PDP-10. The game involved the player moving a character around a cave with simple one- or two-word commands. After each command, the game responded with a textual description of the current location in the cave. "xyzzy" was a magic-word command that, if the character was in the right place in the cave, would "transport" the character to another game location.
Y
Z
- Zero-equivalent
- A value that is treated like 0 (and therefor like false) regardless of the data type. Zero-equivalent values are 0 for integers, 0.0 for floating-point types, false for Booleans, nullptr for pointers, and empty or blank for strings.
- Zero-indexed
- Zero-indexed means that the index values used to access array elements, characters in a string, or data in any linear data structure, begins at 0. Programmers often begin numbering items with 0: the bits in an integer, addresses in memory, bytes on a disk drive, etc.